The JavaScript String match function is used to search a string for a specified pattern and returns an array of all the matches found. The pattern can be a regular expression or a string. If the pattern is a string, it searches for the exact match of the string. If the pattern is a regular expression, it searches for all the matches that fit the pattern. The match function returns null if no match is found. The match function can also be used with the global flag to search for all the matches in a string. Keep reading below to learn how to Javascript String match in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
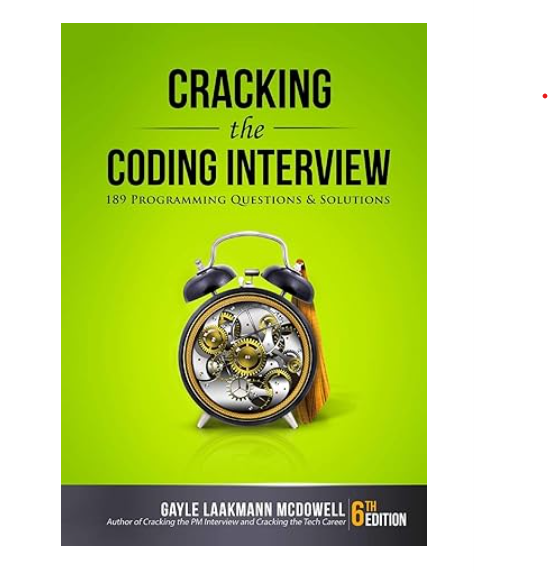
Javascript String match in Java With Example Code
JavaScript String match() method is used to search a string for a specified pattern and returns the matches as an array. In Java, we can use the Pattern and Matcher classes to achieve the same functionality.
To use the Pattern and Matcher classes, we first need to create a Pattern object by compiling a regular expression. The regular expression is the pattern that we want to search for in the string. Once we have the Pattern object, we can create a Matcher object by calling the matcher() method on the Pattern object and passing in the string that we want to search.
Here’s an example code snippet that demonstrates how to use the Pattern and Matcher classes to search for a pattern in a string:
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class StringMatchExample {
public static void main(String[] args) {
String text = "The quick brown fox jumps over the lazy dog";
String pattern = "fox";
Pattern p = Pattern.compile(pattern);
Matcher m = p.matcher(text);
while (m.find()) {
System.out.println("Match found at index " + m.start() + " - " + (m.end() - 1));
}
}
}
In this example, we have a string “The quick brown fox jumps over the lazy dog” and we want to search for the pattern “fox”. We create a Pattern object by compiling the pattern “fox” and then create a Matcher object by calling the matcher() method on the Pattern object and passing in the string “The quick brown fox jumps over the lazy dog”. We then use the find() method on the Matcher object to search for the pattern in the string. The find() method returns true if a match is found and false otherwise. If a match is found, we print out the start and end indices of the match.
In conclusion, we can use the Pattern and Matcher classes in Java to achieve the same functionality as the JavaScript String match() method. By using regular expressions, we can search for patterns in strings and extract the matches as an array.
Equivalent of Javascript String match in Java
In conclusion, the Java programming language provides a powerful and efficient String matching function that is equivalent to the Javascript String match function. The Java String class provides the matches() method, which allows developers to search for a specific pattern within a given string. This method uses regular expressions to match the pattern and returns a boolean value indicating whether the pattern was found or not. The matches() method in Java is a great tool for developers who need to perform complex string matching operations in their applications. It provides a flexible and powerful way to search for patterns within strings, making it an essential tool for any Java developer. Overall, the Java String matches() function is a reliable and efficient alternative to the Javascript String match function. It provides developers with a robust set of tools for working with strings, making it an essential part of any Java developer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |