The JavaScript String match function is used to search a string for a specified pattern and returns an array of all the matches found. The pattern can be a regular expression or a string. If the pattern is a string, it searches for the exact match of the string. If the pattern is a regular expression, it searches for all the matches that fit the pattern. The match function returns null if no match is found. The match function can also be used with the global flag to search for all the matches in a string. Keep reading below to learn how to Javascript String match in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
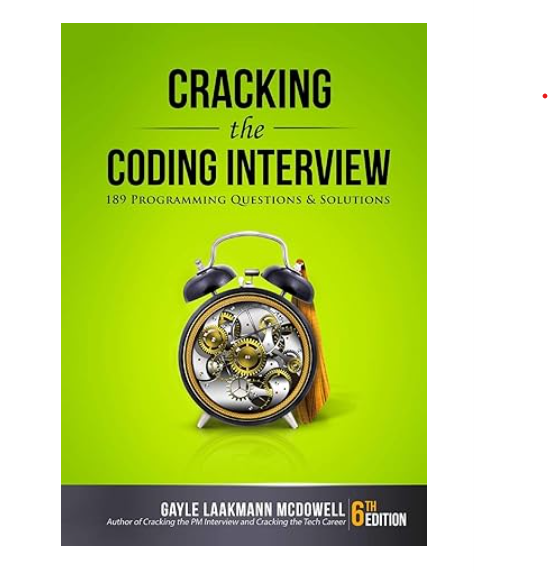
Javascript String match in PHP With Example Code
JavaScript is a popular programming language used for creating interactive web pages. One of the most common tasks in JavaScript is string matching. PHP is another popular programming language used for web development. In this blog post, we will discuss how to use JavaScript string matching in PHP.
To use JavaScript string matching in PHP, we need to use the preg_match function. This function allows us to search a string for a pattern using regular expressions. Regular expressions are a powerful tool for matching patterns in strings.
Here is an example of how to use preg_match to search for a pattern in a string:
$string = "Hello, World!";
$pattern = "/Hello/";
if (preg_match($pattern, $string)) {
echo "Match found!";
} else {
echo "Match not found.";
}
In this example, we are searching for the pattern “Hello” in the string “Hello, World!”. The preg_match function returns true if the pattern is found and false if it is not found.
We can also use regular expressions to search for more complex patterns. For example, we can search for all words that start with the letter “a” using the following pattern:
$string = "apple, banana, avocado";
$pattern = "/\ba\w*/";
preg_match_all($pattern, $string, $matches);
print_r($matches[0]);
In this example, we are using the \b metacharacter to match the beginning of a word and the \w metacharacter to match any word character. The * quantifier matches zero or more occurrences of the preceding character or group.
The preg_match_all function is used to find all matches in the string. The matches are stored in an array, which we can print using the print_r function.
In conclusion, using JavaScript string matching in PHP can be a powerful tool for searching and manipulating strings. By using regular expressions, we can search for complex patterns and extract specific information from strings.
Equivalent of Javascript String match in PHP
In conclusion, the PHP equivalent of the Javascript String match function is the preg_match function. This function allows developers to search for a specific pattern within a string and return the matches found. It also provides additional options for case sensitivity and global matching. While the syntax may differ slightly from the Javascript String match function, the preg_match function is a powerful tool for PHP developers looking to manipulate and search through strings in their code. By understanding the similarities and differences between these two functions, developers can choose the best tool for their specific needs and create efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |