The JavaScript String match function is used to search a string for a specified pattern and returns an array of all the matches found. The pattern can be a regular expression or a string. If the pattern is a string, it searches for the exact match of the string. If the pattern is a regular expression, it searches for all the matches that fit the pattern. The match function returns null if no match is found. The match function can also be used with the global flag to search for all the matches in a string. Keep reading below to learn how to Javascript String match in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
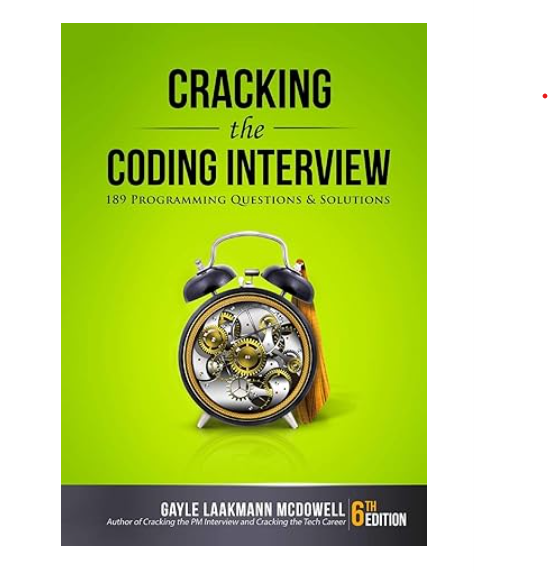
Javascript String match in Rust With Example Code
JavaScript’s `String.match()` method is a powerful tool for finding matches within a string. If you’re working in Rust, you might be wondering how to achieve the same functionality. Fortunately, Rust provides a similar method called `Regex::find()`.
To use `Regex::find()`, you’ll first need to import the `regex` crate. You can do this by adding the following line to your `Cargo.toml` file:
[dependencies]
regex = "1.5.4"
Once you’ve imported the crate, you can use `Regex::find()` to search for matches within a string. Here’s an example:
use regex::Regex;
fn main() {
let re = Regex::new(r"\d+").unwrap();
let text = "The quick brown fox jumps over the lazy dog 42 times.";
let matches: Vec<_> = re.find_iter(text).map(|m| m.as_str()).collect();
println!("{:?}", matches);
}
In this example, we’re searching for any sequence of one or more digits within the string. The `Regex::new()` method creates a new regular expression object, and the `find_iter()` method searches for all matches within the string. We then use the `map()` method to convert each match to a string, and the `collect()` method to collect all matches into a vector.
With `Regex::find()`, you can achieve the same functionality as JavaScript’s `String.match()` method in Rust. Happy coding!
Equivalent of Javascript String match in Rust
In conclusion, the Rust programming language provides a powerful and efficient alternative to the Javascript String match function. With the use of regular expressions and the built-in Regex crate, Rust developers can easily search and match patterns within strings. The Regex crate offers a variety of methods and options for matching, making it a versatile tool for any string manipulation task. Additionally, Rust’s strong type system and memory safety features ensure that code using the Regex crate is reliable and secure. Overall, the equivalent Javascript String match function in Rust is a valuable addition to any developer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |