The JavaScript String replace function is used to replace a specified substring or regular expression pattern in a string with a new substring or value. It takes two parameters: the first parameter is the substring or regular expression pattern to be replaced, and the second parameter is the new substring or value to replace it with. If the first parameter is a regular expression, it can be used to replace all occurrences of the pattern in the string. The replace function returns a new string with the replacements made, leaving the original string unchanged. Keep reading below to learn how to Javascript String replace in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
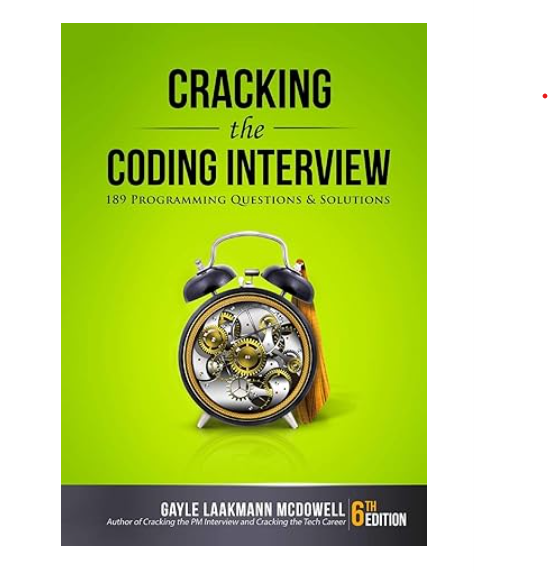
Javascript String replace in C++ With Example Code
JavaScript’s `String.replace()` method is a powerful tool for manipulating strings. However, if you’re working in C++, you might be wondering how to achieve the same functionality. Fortunately, C++ provides a similar method for replacing substrings within a string.
The `std::string::replace()` method allows you to replace a portion of a string with another string. Here’s an example:
std::string str = "Hello, world!";
str.replace(7, 5, "C++");
In this example, we’re replacing the substring “world” (starting at index 7 and with a length of 5 characters) with the string “C++”. The resulting string will be “Hello, C++!”.
You can also use `std::regex_replace()` to replace substrings using regular expressions. Here’s an example:
std::string str = "The quick brown fox jumps over the lazy dog.";
std::regex vowels("[aeiou]");
std::string result = std::regex_replace(str, vowels, "");
In this example, we’re using a regular expression to match all vowels in the string and replace them with an empty string. The resulting string will be “Th qck brwn fx jmps vr th lzy dg.”
With these methods, you can easily replace substrings within a string in C++.
Equivalent of Javascript String replace in C++
In conclusion, the equivalent C++ function for the Javascript String replace function is the std::regex_replace function. This function allows for the replacement of substrings within a string using regular expressions, just like the Javascript function. While the syntax may be slightly different, the functionality is very similar and can be used to achieve the same results. It is important to note that regular expressions can be a powerful tool, but they can also be complex and difficult to understand. Therefore, it is important to use them with caution and to thoroughly test any code that utilizes them. Overall, the std::regex_replace function is a valuable tool for C++ developers who need to manipulate strings and replace substrings within them.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |