The JavaScript String replace function is used to replace a specified value or pattern in a string with a new value. It takes two parameters: the first parameter is the value or pattern to be replaced, and the second parameter is the new value to replace it with. If the first parameter is a regular expression, it can be used to replace multiple occurrences of the pattern in the string. The replace function returns a new string with the replaced values, leaving the original string unchanged. If the first parameter is not found in the string, the function returns the original string. Keep reading below to learn how to Javascript String replace in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
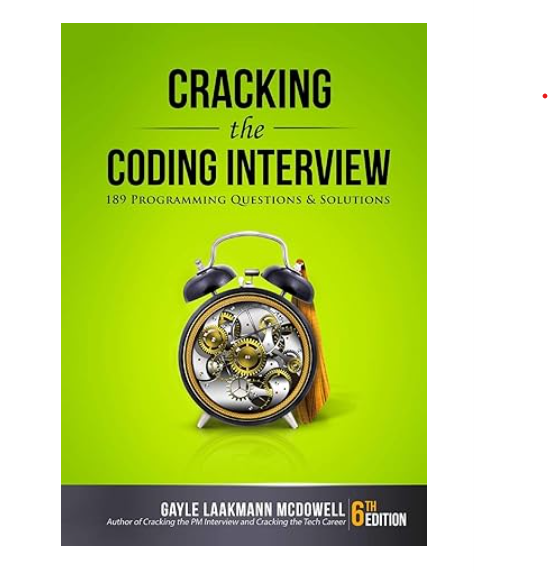
Javascript String replace in Go With Example Code
JavaScript is a popular programming language used for web development. One of its most commonly used methods is the replace()
method, which allows you to replace a specified value in a string with another value. If you’re working with Go, you may be wondering how to perform this same action. In this blog post, we’ll explore how to use the strings.Replace()
function in Go to replace values in a string.
The strings.Replace()
function in Go is similar to the replace()
method in JavaScript. It takes three arguments:
- The original string
- The value to be replaced
- The new value
Here’s an example of how to use the strings.Replace()
function:
package main
import (
"fmt"
"strings"
)
func main() {
originalString := "Hello, World!"
newString := strings.Replace(originalString, "World", "Gopher", -1)
fmt.Println(newString)
}
In this example, we’re replacing the word “World” with “Gopher” in the original string “Hello, World!”. The -1
parameter indicates that we want to replace all occurrences of the value, rather than just the first one.
Using the strings.Replace()
function in Go is a simple and effective way to replace values in a string. Whether you’re working on a small project or a large-scale application, this function can help you manipulate strings with ease.
Equivalent of Javascript String replace in Go
In conclusion, the equivalent Javascript String replace function in Go is a powerful tool for developers who are looking to manipulate strings in their Go programs. With its ability to replace specific characters or patterns within a string, this function can help streamline code and make it more efficient. While there are some differences between the Javascript and Go versions of this function, the basic functionality remains the same. By understanding how to use the replace function in Go, developers can take their string manipulation skills to the next level and create more robust and effective programs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |