The JavaScript String search() function is used to search for a specified string or regular expression within a given string. It returns the index of the first occurrence of the specified search value, or -1 if the search value is not found. The search() function can take a regular expression as its argument, allowing for more complex search patterns. It is a useful tool for finding specific substrings within a larger string and can be used in a variety of applications, such as form validation or data processing. Keep reading below to learn how to Javascript String search in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
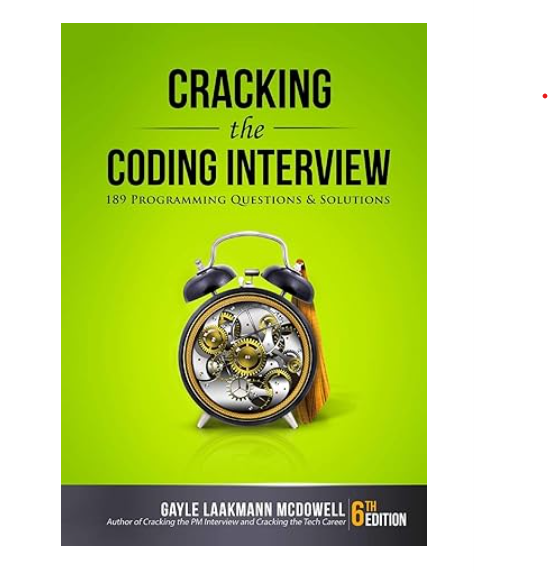
Javascript String search in C# With Example Code
When working with strings in C#, it is often necessary to search for a specific substring within a larger string. This can be accomplished using the string.IndexOf()
method, which returns the index of the first occurrence of the specified substring within the string.
The IndexOf()
method takes a single parameter, which is the substring to search for. It also has an optional second parameter, which specifies the starting index for the search. If this parameter is not specified, the search will begin at the beginning of the string.
Here is an example of using the IndexOf()
method to search for a substring within a string:
string str = "The quick brown fox jumps over the lazy dog";
int index = str.IndexOf("fox");
Console.WriteLine(index); // Output: 16
In this example, the IndexOf()
method is used to search for the substring “fox” within the string “The quick brown fox jumps over the lazy dog”. The method returns the index of the first occurrence of the substring, which is 16.
If the specified substring is not found within the string, the IndexOf()
method returns -1. Here is an example:
string str = "The quick brown fox jumps over the lazy dog";
int index = str.IndexOf("cat");
Console.WriteLine(index); // Output: -1
In this example, the IndexOf()
method is used to search for the substring “cat” within the string “The quick brown fox jumps over the lazy dog”. Since “cat” is not found within the string, the method returns -1.
Equivalent of Javascript String search in C#
In conclusion, the equivalent Javascript String search function in C# is the IndexOf method. This method allows developers to search for a specific substring within a string and returns the index of the first occurrence of the substring. It also provides options for case sensitivity and starting index. While the syntax may differ slightly between the two languages, the functionality remains the same. By understanding the similarities and differences between these two languages, developers can easily transition between them and create efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |