The JavaScript String search() function is used to search for a specified string or regular expression within a given string. It returns the index of the first occurrence of the specified search value, or -1 if the search value is not found. The search() function can take a regular expression as its argument, allowing for more complex search patterns. It is a useful tool for finding specific substrings within a larger string and can be used in a variety of applications, such as form validation or data processing. Keep reading below to learn how to Javascript String search in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
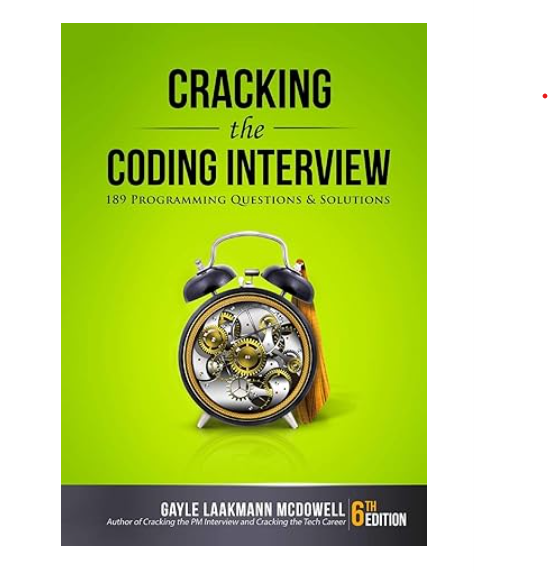
Javascript String search in C++ With Example Code
Searching for a substring within a string is a common task in programming. In C++, you can use the find
function to search for a substring within a string. However, if you want to search for a substring using JavaScript syntax, you can use the string::find
function with a regular expression.
Here’s an example:
std::string str = "Hello, world!";
std::regex pattern("world");
std::smatch match;
if (std::regex_search(str, match, pattern)) {
std::cout << "Found match: " << match.str() << std::endl;
}
In this example, we create a string str
and a regular expression pattern "world"
. We then use the regex_search
function to search for the pattern within the string. If a match is found, we print the matched substring using the match.str()
function.
Keep in mind that regular expressions can be more powerful than simple substring searches. You can use regular expressions to search for patterns within a string, such as matching all email addresses or phone numbers within a block of text.
Equivalent of Javascript String search in C++
In conclusion, the equivalent C++ function for the Javascript String search function is the std::string::find() function. This function allows us to search for a specific substring within a given string and returns the index of the first occurrence of the substring. It is a powerful tool for string manipulation in C++ and can be used in a variety of applications. By understanding the similarities and differences between the Javascript String search function and the C++ std::string::find() function, developers can effectively utilize both languages to create efficient and effective programs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |