The JavaScript String search function is used to search for a specified string within a given string. It returns the index of the first occurrence of the specified string, or -1 if the string is not found. The search function can take a regular expression as an argument, allowing for more complex search patterns. If no argument is provided, the search function returns the index of the first occurrence of the empty string. The search function is case-sensitive, but can be made case-insensitive by using the regular expression flag “i”. Keep reading below to learn how to Javascript String search in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
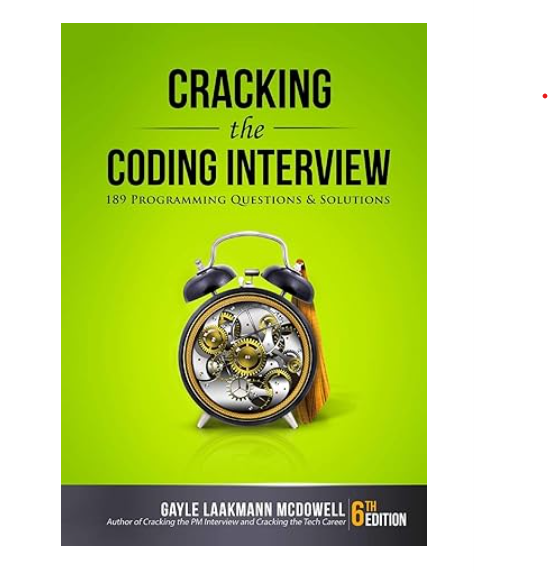
Javascript String search in Go With Example Code
JavaScript String search is a powerful tool that allows you to search for a specific string within a larger string. In Go, you can use the `strings` package to perform similar operations.
To search for a string in Go, you can use the `strings.Contains` function. This function takes two arguments: the string to search in, and the string to search for. It returns a boolean value indicating whether the search string was found.
Here’s an example of how to use `strings.Contains`:
package main
import (
"fmt"
"strings"
)
func main() {
str := "The quick brown fox jumps over the lazy dog"
searchStr := "fox"
if strings.Contains(str, searchStr) {
fmt.Printf("'%s' was found in '%s'\n", searchStr, str)
} else {
fmt.Printf("'%s' was not found in '%s'\n", searchStr, str)
}
}
In this example, we’re searching for the string “fox” within the larger string “The quick brown fox jumps over the lazy dog”. Since “fox” is found within the larger string, the program will output “‘fox’ was found in ‘The quick brown fox jumps over the lazy dog'”.
You can also use `strings.Index` to find the index of the first occurrence of a string within another string. This function takes the same arguments as `strings.Contains`, but returns the index of the first occurrence of the search string within the larger string, or -1 if the search string is not found.
Here’s an example of how to use `strings.Index`:
package main
import (
"fmt"
"strings"
)
func main() {
str := "The quick brown fox jumps over the lazy dog"
searchStr := "fox"
index := strings.Index(str, searchStr)
if index != -1 {
fmt.Printf("'%s' was found at index %d in '%s'\n", searchStr, index, str)
} else {
fmt.Printf("'%s' was not found in '%s'\n", searchStr, str)
}
}
In this example, we’re searching for the string “fox” within the larger string “The quick brown fox jumps over the lazy dog”. Since “fox” is found at index 16 within the larger string, the program will output “‘fox’ was found at index 16 in ‘The quick brown fox jumps over the lazy dog'”.
Overall, the `strings` package in Go provides a powerful set of tools for searching and manipulating strings. By using functions like `strings.Contains` and `strings.Index`, you can easily search for specific strings within larger strings and perform other string operations as needed.
Equivalent of Javascript String search in Go
In conclusion, the equivalent Javascript String search function in Go provides a powerful tool for developers to search for specific patterns within a string. By using the built-in `regexp` package, developers can easily create regular expressions to match their desired pattern and use the `MatchString` function to search for it within a given string. This functionality is particularly useful for tasks such as data validation, text parsing, and pattern matching. With its simplicity and efficiency, the Go String search function is a valuable addition to any developer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |