The JavaScript String search function is used to search for a specified string within a given string. It returns the index of the first occurrence of the specified string, or -1 if the string is not found. The search function can take a regular expression as an argument, allowing for more complex search patterns. If no argument is provided, the search function returns the index of the first occurrence of the empty string. The search function is case-sensitive, but can be made case-insensitive by using the regular expression flag “i”. Keep reading below to learn how to Javascript String search in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
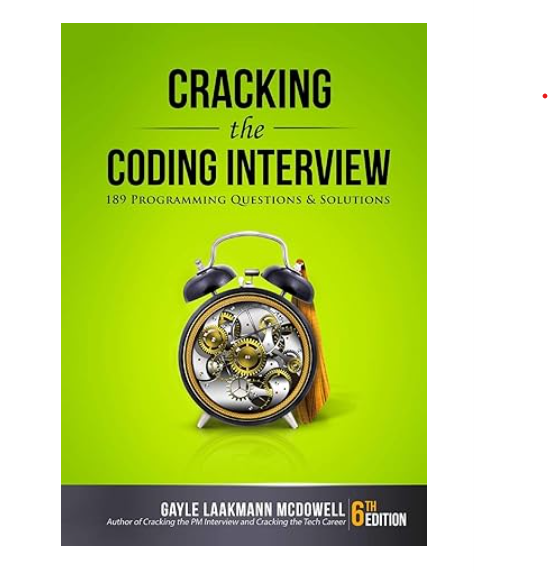
Javascript String search in Kotlin With Example Code
JavaScript String search is a powerful tool that allows you to search for a specific string within a larger string. In Kotlin, you can use the built-in `contains` function to achieve the same result.
To use the `contains` function, simply call it on a string and pass in the string you want to search for as an argument. The function will return a boolean value indicating whether or not the string contains the search term.
Here’s an example:
val myString = "Hello, world!"
val searchTerm = "world"
if (myString.contains(searchTerm)) {
println("Found it!")
} else {
println("Not found.")
}
In this example, we’re searching for the string “world” within the larger string “Hello, world!”. Since the string contains the search term, the program will output “Found it!”.
You can also use the `contains` function to perform case-insensitive searches by passing in the `ignoreCase` parameter:
val myString = "Hello, world!"
val searchTerm = "WORLD"
if (myString.contains(searchTerm, ignoreCase = true)) {
println("Found it!")
} else {
println("Not found.")
}
In this example, we’re searching for the string “WORLD” within the larger string “Hello, world!”, but we’re ignoring case. Since the string contains the search term (even though the case doesn’t match), the program will output “Found it!”.
Overall, the `contains` function is a simple and effective way to search for strings in Kotlin.
Equivalent of Javascript String search in Kotlin
In conclusion, Kotlin provides a powerful and efficient way to search for substrings within a string using the `contains()` function. This function is equivalent to the `includes()` function in JavaScript and allows developers to easily check if a string contains a specific substring. Additionally, Kotlin also provides other useful string manipulation functions such as `startsWith()`, `endsWith()`, and `indexOf()` that can be used to perform more complex string operations. With these tools at their disposal, Kotlin developers can easily manipulate and search through strings to build robust and efficient applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |