The JavaScript String slice function is used to extract a portion of a string and return it as a new string. It takes two parameters: the starting index and the ending index (optional). The starting index is the position of the first character to be included in the new string, while the ending index is the position of the first character to be excluded. If the ending index is not specified, the slice function will extract all characters from the starting index to the end of the string. The original string is not modified by the slice function. Keep reading below to learn how to Javascript String slice in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
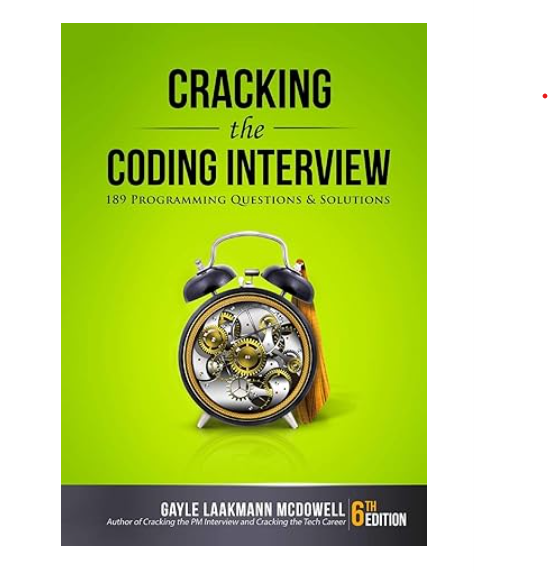
Javascript String slice in Go With Example Code
JavaScript’s `slice()` method is a commonly used function to extract a portion of a string. In Go, we can achieve the same functionality using the `slice` function from the `strings` package.
To use the `slice` function, we need to pass in the string we want to extract a portion of, and the starting and ending indices of the portion we want to extract. The starting index is inclusive, while the ending index is exclusive.
Here’s an example of how to use the `slice` function in Go:
package main
import (
"fmt"
"strings"
)
func main() {
str := "Hello, world!"
portion := strings.Slice(str, 0, 5)
fmt.Println(portion)
}
In this example, we’re extracting the first 5 characters of the string “Hello, world!”. The `Slice` function returns a new string containing the extracted portion.
It’s important to note that the `slice` function in Go works similarly to the `slice()` method in JavaScript, but there are some differences in syntax and behavior. For example, in JavaScript, if we pass in a negative index to `slice()`, it will count from the end of the string. In Go, negative indices are not supported, and will result in a runtime error.
Overall, the `slice` function in Go provides a simple and efficient way to extract portions of strings, and can be a useful tool in your programming toolkit.
Equivalent of Javascript String slice in Go
In conclusion, the equivalent function of Javascript’s String slice in Go is the `string` package’s `substring` function. This function allows developers to extract a portion of a string based on the starting and ending indices. It is a useful tool for manipulating strings in Go and can be used in a variety of applications. By understanding the similarities and differences between the two functions, developers can easily transition between the two languages and create efficient and effective code. Overall, the `substring` function is a valuable addition to the Go language and is a great alternative to the String slice function in Javascript.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |