The JavaScript String slice function is used to extract a portion of a string and return it as a new string. It takes two parameters: the starting index and the ending index (optional). The starting index is the position of the first character to be included in the new string, while the ending index is the position of the first character to be excluded. If the ending index is not specified, the slice function will extract all characters from the starting index to the end of the string. The original string is not modified by the slice function. Keep reading below to learn how to Javascript String slice in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
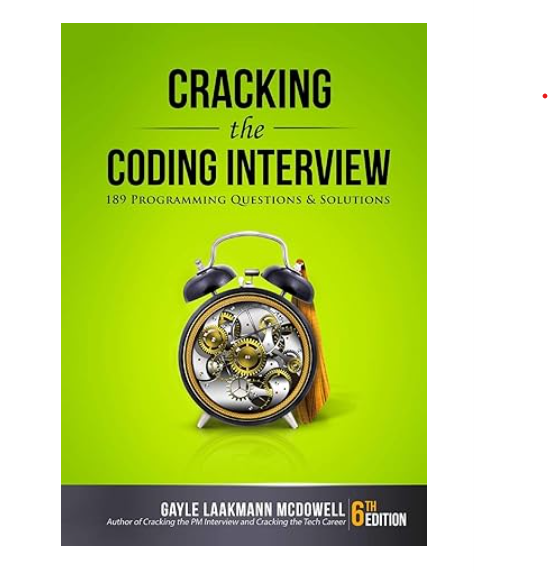
Javascript String slice in Kotlin With Example Code
JavaScript’s slice()
method is a useful tool for manipulating strings. In Kotlin, you can achieve the same functionality using the substring()
method.
The substring()
method takes two arguments: the starting index and the ending index. The starting index is inclusive, while the ending index is exclusive. This means that the character at the starting index will be included in the substring, but the character at the ending index will not.
Here’s an example:
val str = "Hello, world!"
val slicedStr = str.substring(0, 5)
println(slicedStr) // Output: "Hello"
In this example, we’re creating a new string called slicedStr
that contains the first five characters of the original string, str
.
If you want to slice a string from a specific index to the end of the string, you can omit the second argument:
val str = "Hello, world!"
val slicedStr = str.substring(7)
println(slicedStr) // Output: "world!"
In this example, we’re creating a new string called slicedStr
that contains all the characters of the original string from index 7 to the end.
Using the substring()
method, you can easily slice and manipulate strings in Kotlin.
Equivalent of Javascript String slice in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to manipulate strings using the slice function. This function is equivalent to the JavaScript String slice function and allows developers to extract a portion of a string based on a specified range of indices. With its simple syntax and flexibility, the slice function in Kotlin is a valuable tool for any developer working with strings. Whether you’re building a web application or a mobile app, the slice function in Kotlin can help you streamline your code and improve the performance of your application. So, if you’re looking for a reliable and efficient way to manipulate strings in Kotlin, be sure to give the slice function a try.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |