The JavaScript String slice function is used to extract a portion of a string and return it as a new string. It takes two parameters: the starting index and the ending index (optional). The starting index is the position of the first character to be included in the new string, while the ending index is the position of the first character to be excluded. If the ending index is not specified, the slice function will extract all characters from the starting index to the end of the string. The original string is not modified by the slice function. Keep reading below to learn how to Javascript String slice in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
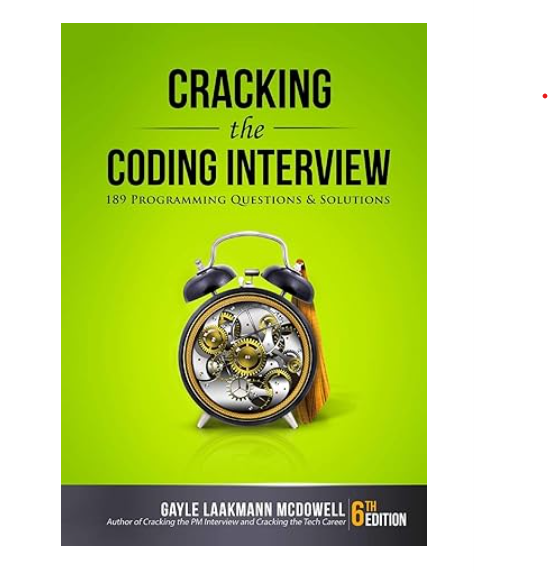
Javascript String slice in Rust With Example Code
JavaScript developers who are looking to learn Rust may be interested in how to perform a string slice in Rust. In JavaScript, the `slice()` method is used to extract a section of a string and return it as a new string. Rust has a similar method called `slice()` that can be used to achieve the same result.
To use the `slice()` method in Rust, you first need to create a string. This can be done using the `String::from()` method:
let my_string = String::from("Hello, world!");
Once you have a string, you can use the `slice()` method to extract a section of it. The `slice()` method takes two arguments: the starting index and the ending index. The starting index is inclusive, while the ending index is exclusive. This means that the character at the starting index will be included in the slice, but the character at the ending index will not.
let my_slice = &my_string[0..5];
In this example, the slice will start at index 0 (the first character) and end at index 5 (the sixth character). This will result in a new string that contains the first five characters of the original string: “Hello”.
It’s important to note that the `slice()` method does not modify the original string. Instead, it returns a new string that contains the extracted section.
In conclusion, performing a string slice in Rust is similar to performing one in JavaScript. By using the `slice()` method, you can extract a section of a string and return it as a new string.
Equivalent of Javascript String slice in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to manipulate strings using the slice function. This function is equivalent to the JavaScript String slice function and allows developers to extract substrings from a string based on a specified range of indices. With Rust’s strong type system and memory safety features, developers can confidently use the slice function to manipulate strings without worrying about common errors such as buffer overflows or null pointer exceptions. Overall, the slice function in Rust is a valuable tool for developers who want to work with strings in a safe and efficient manner.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |