The JavaScript String substr() function is used to extract a portion of a string, starting from a specified index position and continuing for a specified number of characters. The function takes two arguments: the starting index position and the length of the substring to be extracted. If the length argument is not provided, the function will extract the remaining characters from the starting index position to the end of the string. The substr() function does not modify the original string, but returns a new string containing the extracted substring. Keep reading below to learn how to Javascript String substr in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
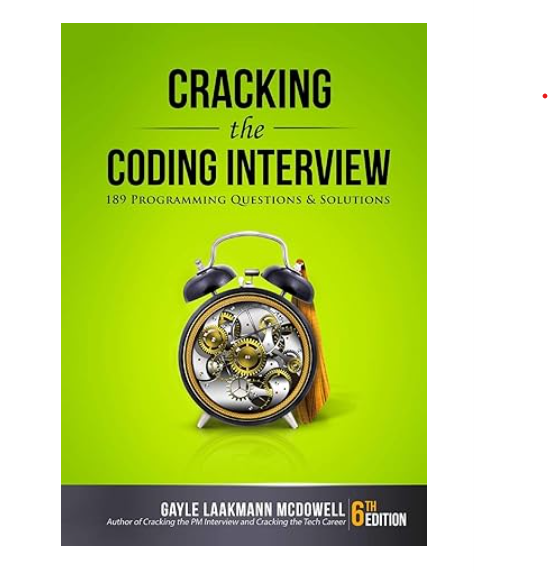
Javascript String substr in C++ With Example Code
JavaScript’s substr()
method is used to extract a portion of a string. In C++, the equivalent function is substr()
which is a member function of the std::string
class.
The syntax for using substr()
in C++ is:
string substr (size_t pos, size_t len) const;
The substr()
function takes two arguments:
pos
: The starting position of the substring. This is a zero-based index.len
: The length of the substring to be extracted.
Here’s an example of how to use substr()
in C++:
#include <iostream>
#include <string>
using namespace std;
int main () {
string str = "Hello, World!";
string substr = str.substr (7, 5);
cout << substr;
return 0;
}
In this example, the substr()
function is used to extract the substring “World” from the string “Hello, World!”. The starting position of the substring is 7 (which is the index of the letter “W” in the string), and the length of the substring is 5 (which is the number of characters in the word “World”).
Equivalent of Javascript String substr in C++
In conclusion, the C++ language provides a powerful and efficient way to manipulate strings using the substr function. This function allows developers to extract a portion of a string based on a specified starting index and length. The equivalent of the Javascript String substr function in C++ provides a similar functionality, but with the added benefit of being able to work with strings in a more low-level and performant way. Whether you are working on a small project or a large-scale application, the substr function in C++ is a valuable tool for string manipulation.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |