The JavaScript String substr() function is used to extract a portion of a string, starting from a specified index position and continuing for a specified number of characters. The function takes two arguments: the starting index position and the length of the substring to be extracted. If the length argument is not provided, the function will extract the remaining characters from the starting index position to the end of the string. The substr() function does not modify the original string, but returns a new string containing the extracted substring. Keep reading below to learn how to Javascript String substr in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
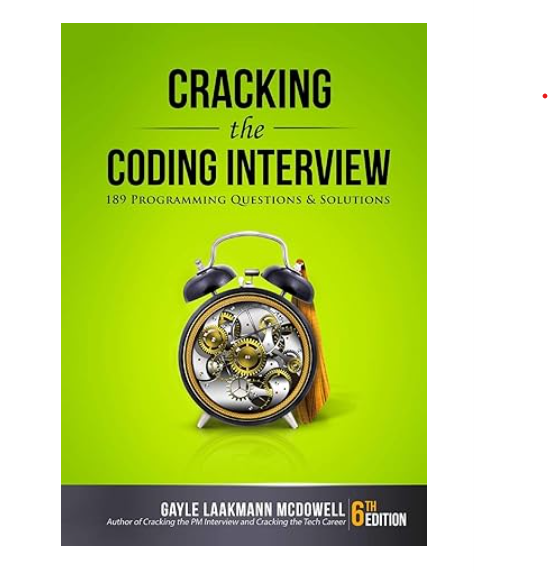
Javascript String substr in Go With Example Code
JavaScript’s `substr()` method is used to extract a portion of a string. In Go, we can achieve the same functionality using the `substring` method of the `strings` package.
To use the `substring` method, we need to pass the starting index and the length of the substring we want to extract. Here’s an example:
package main
import (
"fmt"
"strings"
)
func main() {
str := "Hello, World!"
substr := strings.Substring(str, 7, 5)
fmt.Println(substr)
}
In this example, we’re extracting a substring starting from index 7 with a length of 5 characters. The output of this program will be `World`.
It’s important to note that the starting index is zero-based, meaning the first character of the string has an index of 0. Also, if the length of the substring is not specified, the method will extract the rest of the string starting from the specified index.
In conclusion, the `substring` method of the `strings` package in Go can be used to achieve the same functionality as JavaScript’s `substr()` method.
Equivalent of Javascript String substr in Go
In conclusion, the Go programming language provides a powerful and efficient way to manipulate strings using the `substring` function. This function is similar to the `substr` function in JavaScript, allowing developers to extract a portion of a string based on a starting index and a length. With its simple syntax and high performance, the `substring` function in Go is a valuable tool for any developer working with strings. Whether you’re building a web application or a command-line tool, the `substring` function in Go can help you extract the data you need from your strings quickly and easily. So, if you’re looking for a reliable and efficient way to manipulate strings in Go, be sure to check out the `substring` function and see how it can help you streamline your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |