The JavaScript String substr() function is used to extract a portion of a string, starting from a specified index position and continuing for a specified number of characters. The function takes two arguments: the starting index position and the length of the substring to be extracted. If the length argument is not provided, the function will extract the remaining characters from the starting index position to the end of the string. The substr() function does not modify the original string, but returns a new string containing the extracted substring. This function is useful for manipulating and extracting specific parts of a string in JavaScript. Keep reading below to learn how to Javascript String substr in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
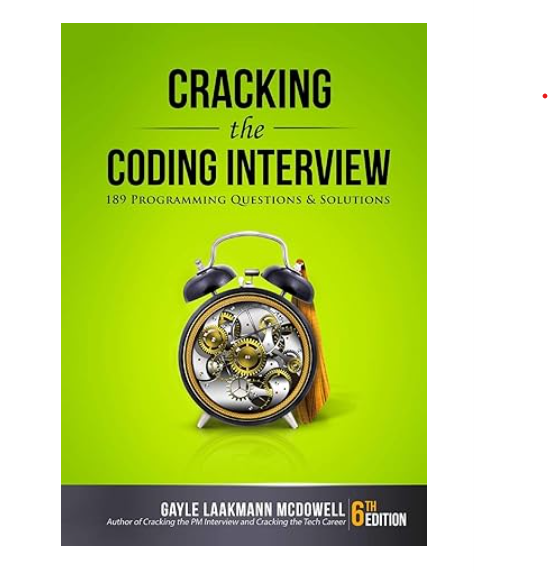
Javascript String substr in Rust With Example Code
JavaScript’s `substr()` method is used to extract a part of a string. Rust provides a similar method called `get()` to achieve the same functionality. In this blog post, we will explore how to use `get()` to extract substrings in Rust.
To use `get()`, we need to provide the starting index and the length of the substring we want to extract. Here’s an example:
let my_string = "Hello, world!";
let my_substring = &my_string[0..5];
println!("{}", my_substring); // Output: "Hello"
In the example above, we created a string `my_string` and used the `get()` method to extract the first 5 characters of the string. We then printed the extracted substring to the console.
We can also use variables to specify the starting index and length of the substring. Here’s an example:
let my_string = "Hello, world!";
let start_index = 7;
let length = 5;
let my_substring = &my_string[start_index..(start_index + length)];
println!("{}", my_substring); // Output: "world"
In the example above, we used variables `start_index` and `length` to specify the starting index and length of the substring we want to extract. We then used these variables in the `get()` method to extract the substring “world” from the original string.
In conclusion, Rust’s `get()` method provides a simple and efficient way to extract substrings from strings. By providing the starting index and length of the substring, we can easily extract the desired portion of the string.
Equivalent of Javascript String substr in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to manipulate strings using its built-in string functions. One of these functions is the equivalent of the Javascript String substr function, which allows developers to extract a portion of a string based on a specified starting index and length. This function is easy to use and provides a lot of flexibility in string manipulation, making it a valuable tool for Rust developers. With its focus on performance and safety, Rust is quickly becoming a popular choice for building high-performance applications, and its string manipulation capabilities are just one of the many reasons why. Whether you’re a seasoned developer or just starting out, Rust’s string functions are definitely worth exploring.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |