The JavaScript String substring() function is used to extract a portion of a string and return it as a new string. It takes two parameters: the starting index and the ending index of the substring. The starting index is inclusive, meaning the character at that index is included in the substring, while the ending index is exclusive, meaning the character at that index is not included in the substring. If the ending index is not specified, the substring will include all characters from the starting index to the end of the string. If the starting index is greater than the ending index, the substring function will swap the two values before extracting the substring. Keep reading below to learn how to Javascript String substring in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
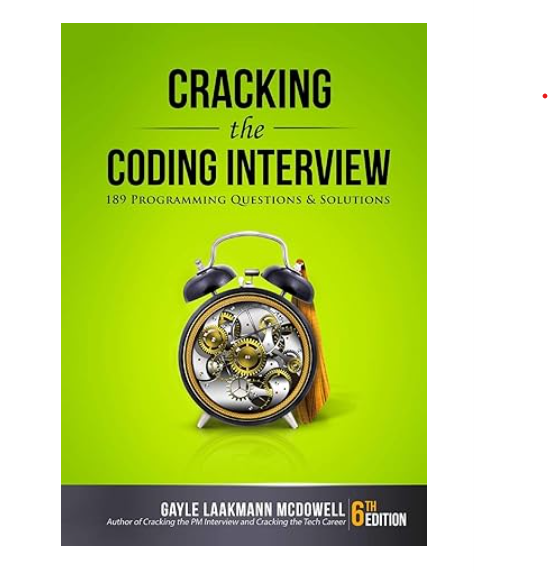
Javascript String substring in Rust With Example Code
JavaScript’s `substring()` method is used to extract a portion of a string. Rust, being a systems programming language, does not have a built-in method for this. However, we can use Rust’s string slicing syntax to achieve the same result.
To extract a substring from a Rust string, we can use the slicing syntax `string[start..end]`. This will return a new string that contains the characters from the `start` index up to, but not including, the `end` index.
Here’s an example of how to use string slicing to extract a substring in Rust:
let my_string = "Hello, world!";
let substring = &my_string[0..5];
println!("{}", substring); // Output: "Hello"
In this example, we create a string `my_string` and then use slicing syntax to extract the first five characters of the string, which is “Hello”. We then print the substring to the console.
It’s important to note that when using string slicing in Rust, the `end` index is exclusive. This means that the character at the `end` index is not included in the resulting substring.
In conclusion, while Rust does not have a built-in `substring()` method like JavaScript, we can use string slicing syntax to achieve the same result.
Equivalent of Javascript String substring in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to manipulate strings using its built-in substring function. This function, similar to the JavaScript String substring function, allows developers to extract a portion of a string based on a specified starting and ending index. With Rust’s focus on performance and safety, this substring function is optimized to handle large strings and prevent common errors such as buffer overflows. Whether you are a seasoned Rust developer or just starting out, the substring function is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |