The toLocaleLowerCase() function in JavaScript is used to convert a string to lowercase letters based on the user’s locale. This means that the function will take into account the language and region settings of the user’s computer or device and convert the string to lowercase accordingly. The function does not modify the original string, but instead returns a new string with all the characters converted to lowercase. This function is useful when working with strings that may contain characters with different case sensitivity rules in different languages. Keep reading below to learn how to Javascript String toLocaleLowerCase in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
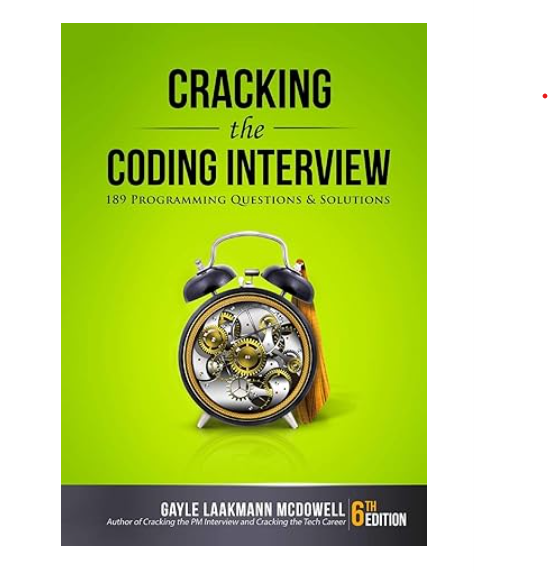
Javascript String toLocaleLowerCase in Go With Example Code
JavaScript’s `toLocaleLowerCase()` method is used to convert a string to lowercase based on the host environment’s current locale. In Go, we can achieve the same functionality using the `golang.org/x/text` package.
To use this package, we first need to install it using the following command:
go get golang.org/x/text
Once the package is installed, we can use the `ToLower` function from the `unicode` package to convert a string to lowercase based on a specific locale. Here’s an example:
import (
"fmt"
"golang.org/x/text/unicode"
)
func main() {
str := "HELLO WORLD"
locale := "en-US"
toLower := unicode.ToLower(unicode.TurkishCase, str)
fmt.Println(toLower)
}
In this example, we’re converting the string “HELLO WORLD” to lowercase based on the Turkish locale. The output of this program will be “hello world”.
By using the `golang.org/x/text` package, we can easily convert strings to lowercase based on any locale. This can be useful when working with multilingual applications or when dealing with user input that may be in different languages.
Equivalent of Javascript String toLocaleLowerCase in Go
In conclusion, the Go programming language provides a powerful and efficient way to manipulate strings using its built-in functions. One such function is the ToLower() function, which converts all the characters in a string to lowercase. However, if you need to convert a string to lowercase based on the rules of a specific locale, you can use the ToLower() function in combination with the strings package’s ToLowerSpecial() function. This function takes a locale as an argument and returns a new string with all the characters converted to lowercase based on the rules of that locale. With this functionality, Go provides a robust solution for handling string manipulation in a variety of contexts.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |