The toLocaleUpperCase() function in JavaScript is used to convert the characters in a string to uppercase, based on the rules of the specified locale. This function takes an optional parameter that specifies the locale to use for the conversion. If no locale is specified, the default locale of the user’s browser is used. The toLocaleUpperCase() function does not modify the original string, but instead returns a new string with the converted characters. This function is useful for displaying text in a consistent and culturally appropriate way, especially when dealing with multilingual applications. Keep reading below to learn how to Javascript String toLocaleUpperCase in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
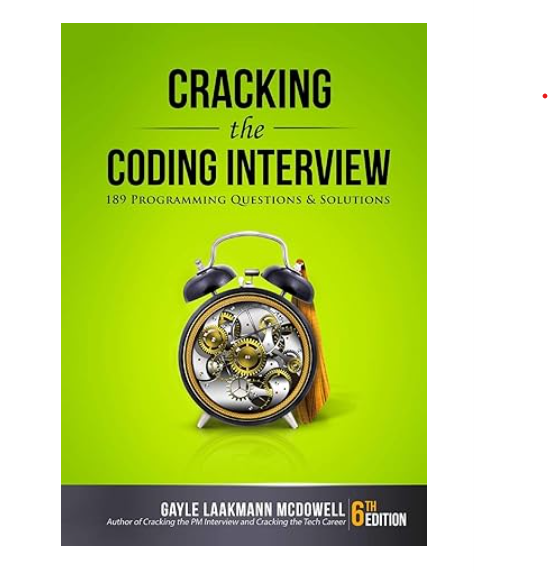
Javascript String toLocaleUpperCase in Go With Example Code
JavaScript’s `toLocaleUpperCase()` method is a useful tool for converting strings to uppercase based on the user’s locale. However, if you’re working with Go, you may be wondering how to achieve the same functionality. Fortunately, Go’s `text/transform` package provides a solution.
To use `text/transform` to convert a string to uppercase based on the user’s locale, you’ll need to first create a `transform.Transformer` object using the `transform.NewReader()` function. This function takes two arguments: the input reader and the `transform.Transformer` object.
“`go
import (
“golang.org/x/text/transform”
“golang.org/x/text/unicode/norm”
)
func toLocaleUpperCase(s string) (string, error) {
t := transform.Chain(norm.NFD, transform.RemoveFunc(isMn), norm.NFC, transform.ToUpper)
output := &strings.Builder{}
input := strings.NewReader(s)
_, err := io.Copy(output, transform.NewReader(input, t))
if err != nil {
return “”, err
}
return output.String(), nil
}
func isMn(r rune) bool {
return unicode.Is(unicode.Mn, r)
}
“`
In the above example, we define a `toLocaleUpperCase()` function that takes a string as input and returns a string and an error. The function first creates a `transform.Chain` object that specifies the transformations to be applied to the input string. In this case, we’re using the `norm.NFD` and `norm.NFC` functions to normalize the string, the `transform.RemoveFunc()` function to remove any combining marks, and the `transform.ToUpper` function to convert the string to uppercase.
Next, we create a `strings.Builder` object to hold the output string, and a `strings.NewReader` object to read the input string. We then use the `transform.NewReader()` function to create a `transform.Reader` object that applies the transformations specified in the `transform.Chain` object.
Finally, we use the `io.Copy()` function to copy the transformed input string to the output string, and return the output string and any errors.
With this function, you can easily convert strings to uppercase based on the user’s locale in your Go applications.
Equivalent of Javascript String toLocaleUpperCase in Go
In conclusion, the Go programming language provides a powerful and efficient way to convert strings to uppercase using the `strings.ToUpper()` function. This function is similar to the `toLocaleUpperCase()` function in JavaScript, but it does not take into account the user’s locale. Instead, it simply converts all characters in the string to uppercase, regardless of their language or cultural context. This makes it a reliable and consistent way to handle string manipulation in Go, and it is a valuable tool for developers who need to work with text data in their applications. Overall, the `strings.ToUpper()` function is a great example of the simplicity and elegance of the Go language, and it is a useful tool for any developer working with strings in their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |