The toLowerCase() function in JavaScript is a built-in method that converts all the characters in a string to lowercase. This function does not modify the original string, but instead returns a new string with all the characters in lowercase. The toLowerCase() function is useful when comparing strings, as it ensures that the comparison is case-insensitive. It can also be used to normalize user input, as it ensures that all characters are in the same case. The syntax for using the toLowerCase() function is as follows: string.toLowerCase(). Keep reading below to learn how to Javascript String toLowerCase in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
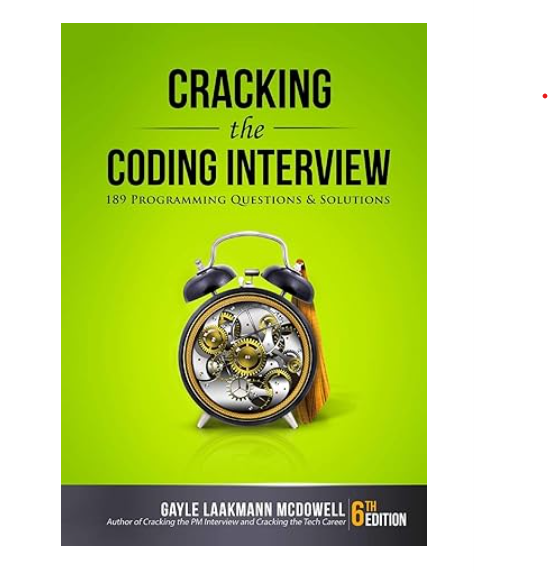
Javascript String toLowerCase in Go With Example Code
JavaScript’s toLowerCase() method is a useful tool for converting strings to lowercase. However, if you’re working in Go, you may be wondering how to achieve the same result. Fortunately, Go provides a simple solution for converting strings to lowercase.
To convert a string to lowercase in Go, you can use the strings.ToLower() function. This function takes a string as its argument and returns a new string with all characters converted to lowercase.
Here’s an example of how to use strings.ToLower() in Go:
package main
import (
"fmt"
"strings"
)
func main() {
str := "HELLO, WORLD!"
fmt.Println(strings.ToLower(str))
}
In this example, we first import the “strings” package, which provides the ToLower() function. We then define a string variable called “str” and assign it the value “HELLO, WORLD!”. Finally, we call strings.ToLower() on “str” and print the result to the console.
When you run this code, you should see the output “hello, world!” printed to the console.
In conclusion, converting a string to lowercase in Go is a simple task thanks to the strings.ToLower() function. By using this function, you can easily manipulate strings in your Go programs.
Equivalent of Javascript String toLowerCase in Go
In conclusion, the Go programming language provides a powerful and efficient way to manipulate strings with its built-in functions. One of these functions is the equivalent of the JavaScript String toLowerCase function, which allows developers to convert all uppercase characters in a string to lowercase. This function is easy to use and can be applied to any string variable in Go. By using this function, developers can ensure that their code is consistent and easy to read, making it easier to maintain and debug. Overall, the toLowerCase function in Go is a valuable tool for any developer working with strings in their code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |