The JavaScript String toString() function is used to convert a string object to a string primitive. It returns a string representation of the object on which it is called. If the object is already a string primitive, the function simply returns the object. This function is useful when you need to convert a string object to a string primitive so that you can perform string operations on it. It is also commonly used to convert other data types to strings, such as numbers or booleans. Keep reading below to learn how to Javascript String toString in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
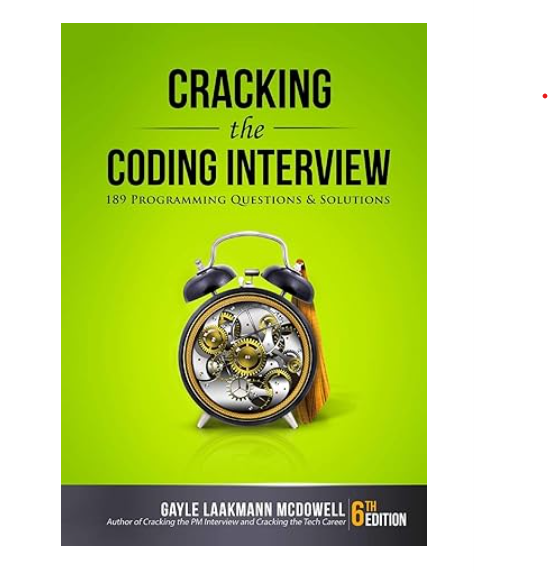
Javascript String toString in Rust With Example Code
JavaScript’s `toString()` method is a commonly used function that converts a value to a string. In Rust, there is no direct equivalent to this method, but there are several ways to achieve the same result.
One way to convert a value to a string in Rust is to use the `format!()` macro. This macro allows you to format a string with variables, similar to how you would use `printf()` in C. Here’s an example:
let my_number = 42;
let my_string = format!("{}", my_number);
In this example, we use the `format!()` macro to create a string that contains the value of `my_number`. The `{}` is a placeholder for the value, and the value is passed as an argument to the macro.
Another way to convert a value to a string in Rust is to use the `to_string()` method. This method is available on many types in Rust, including integers, floats, and booleans. Here’s an example:
let my_number = 42;
let my_string = my_number.to_string();
In this example, we use the `to_string()` method to convert `my_number` to a string.
Overall, while Rust doesn’t have a direct equivalent to JavaScript’s `toString()` method, there are several ways to achieve the same result. The `format!()` macro and the `to_string()` method are two common approaches.
Equivalent of Javascript String toString in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to work with strings. The equivalent of the JavaScript String toString function in Rust is the to_string method. This method allows developers to convert any data type into a string, making it easier to work with and manipulate. Additionally, Rust’s strong type system ensures that the resulting string is always valid and safe to use. Whether you’re building a web application or a system-level program, Rust’s string handling capabilities are sure to make your development process smoother and more efficient. So, if you’re looking for a language that can handle strings with ease, Rust is definitely worth considering.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |