The JavaScript String toUpperCase() function is a built-in method that converts all the characters in a string to uppercase letters. It returns a new string with all the alphabetic characters in uppercase format. This function is useful when you want to standardize the case of a string for comparison or display purposes. The toUpperCase() function does not modify the original string, but instead creates a new string with the uppercase characters. It can be called on any string variable or string literal and takes no arguments. Keep reading below to learn how to Javascript String toUpperCase in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
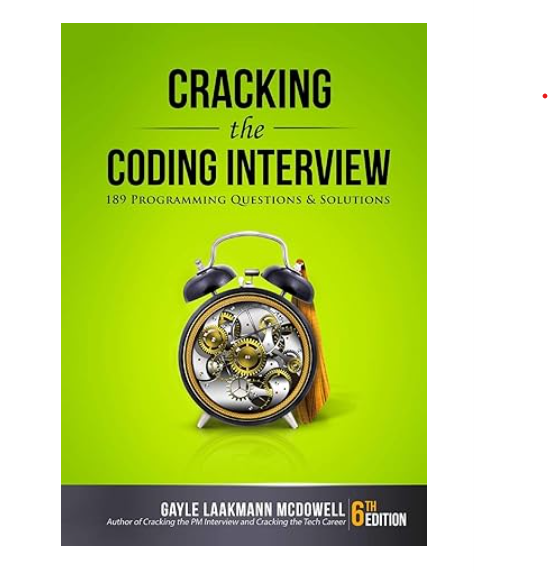
Javascript String toUpperCase in C++ With Example Code
Converting a string to uppercase is a common task in programming. In C++, you can use the std::transform
function to convert a string to uppercase. Here’s an example:
#include <algorithm>
#include <cctype>
#include <string>
#include <iostream>
int main() {
std::string str = "hello world";
std::transform(str.begin(), str.end(), str.begin(), [](unsigned char c){ return std::toupper(c); });
std::cout << str << std::endl;
return 0;
}
In this example, we first define a string str
with the value “hello world”. We then use std::transform
to convert each character in the string to uppercase. The lambda function passed to std::transform
takes an unsigned char as input and returns the uppercase version of that character using the std::toupper
function. Finally, we output the modified string to the console.
Equivalent of Javascript String toUpperCase in C++
In conclusion, the C++ programming language provides a powerful and efficient way to manipulate strings using the toUpperCase() function. This function is equivalent to the JavaScript String toUpperCase() function and allows developers to easily convert all characters in a string to uppercase. By using this function, C++ programmers can ensure that their code is more readable and consistent, making it easier to maintain and debug. Whether you are a seasoned C++ developer or just starting out, the toUpperCase() function is a valuable tool that can help you create more robust and reliable applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |