The JavaScript String valueOf() function returns the primitive value of a String object. This function is automatically called by JavaScript whenever a string object is used in a context where a primitive value is expected, such as when using the “+” operator to concatenate strings. The valueOf() function simply returns the string value of the object, which is the same as the original string that was used to create the object. This function is useful when you need to convert a string object back to its primitive value for further processing or manipulation. Keep reading below to learn how to Javascript String valueOf in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
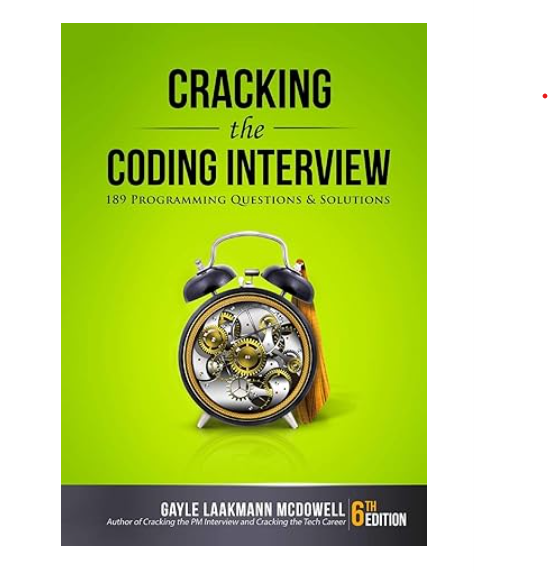
Javascript String valueOf in C++ With Example Code
JavaScript’s `valueOf()` method returns the primitive value of a `String` object. In C++, we can achieve similar functionality using the `c_str()` method of the `std::string` class.
The `c_str()` method returns a pointer to a null-terminated character array that represents the contents of the string. This pointer can be used to access the string’s value as a C-style string.
Here’s an example of how to use `c_str()` to get the primitive value of a `std::string` object:
#include
#include
int main() {
std::string myString = "Hello, world!";
const char* myCString = myString.c_str();
std::cout << myCString << std::endl;
return 0;
}
In this example, we create a `std::string` object called `myString` with the value "Hello, world!". We then use the `c_str()` method to get a pointer to a null-terminated character array that represents the contents of `myString`. Finally, we print the value of `myCString` to the console using `std::cout`.
By using `c_str()`, we can easily get the primitive value of a `std::string` object in C++.
Equivalent of Javascript String valueOf in C++
In conclusion, the equivalent function of the Javascript String valueOf() in C++ is the str() function. Both functions serve the same purpose of returning the string representation of an object. However, it is important to note that C++ is a statically typed language, which means that the type of the object must be explicitly defined before calling the str() function. Additionally, C++ offers a wide range of string manipulation functions that can be used in conjunction with the str() function to perform various operations on strings. Overall, the str() function is a powerful tool in C++ for working with strings and can be used to simplify and streamline string manipulation tasks.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |