The JavaScript String valueOf() function returns the primitive value of a String object. This function is automatically called by JavaScript whenever a string object is used in a context where a primitive value is expected, such as when using the “+” operator to concatenate strings. The valueOf() function simply returns the string value of the object, which is the same as the original string that was used to create the object. This function is useful when you need to convert a string object back to its primitive value for further processing or manipulation. Keep reading below to learn how to Javascript String valueOf in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
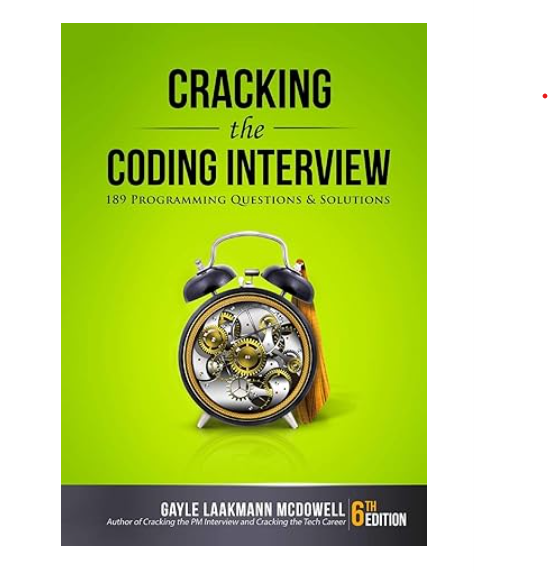
Javascript String valueOf in Rust With Example Code
JavaScript’s `valueOf()` method returns the primitive value of a `String` object. In Rust, we can achieve similar functionality using the `to_string()` method.
To convert a Rust `String` to its primitive value, we can simply call the `to_string()` method on the `String` object. This will return a `String` representation of the object, which can then be converted to a primitive value using Rust’s built-in type conversion functions.
Here’s an example of how to use `to_string()` to convert a Rust `String` to a primitive value:
let my_string = String::from("Hello, world!");
let primitive_value = my_string.to_string().parse::
In this example, we first create a `String` object called `my_string` with the value “Hello, world!”. We then call the `to_string()` method on `my_string` to convert it to a `String` representation. Finally, we use Rust’s `parse()` method to convert the `String` to an `i32` primitive value.
By using Rust’s `to_string()` method and built-in type conversion functions, we can achieve similar functionality to JavaScript’s `valueOf()` method.
Equivalent of Javascript String valueOf in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to work with strings through its String type. The equivalent of the Javascript String valueOf function in Rust is the to_string method, which converts any type that implements the Display trait into a String. This method is easy to use and provides a flexible way to convert data into a string format. Additionally, Rust’s ownership and borrowing system ensures that string manipulation is safe and efficient. Overall, Rust’s String type and to_string method make it a great choice for developers who need to work with strings in their applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |