The Linux “df” command is used to display the amount of disk space available on a file system. It shows the total size of the file system, the amount of space used, the amount of space available, and the percentage of space used. The command can be used with various options to display the information in different formats and to filter the output based on specific criteria. It is a useful tool for monitoring disk usage and identifying potential storage issues.. Keep reading below to learn how to linux df in python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
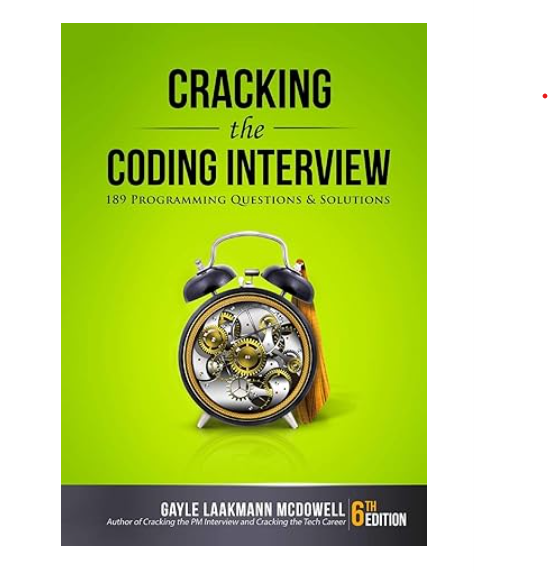
Linux ‘df’ in Python With Example Code
If you’re working with Linux and Python, you may find yourself needing to use the “df” command to check disk space usage. Fortunately, it’s easy to use this command in Python using the “subprocess” module.
First, you’ll need to import the subprocess module:
import subprocess
Next, you can use the “subprocess.run()” method to run the “df” command and capture its output:
result = subprocess.run(['df'], stdout=subprocess.PIPE)
The “stdout” argument tells the “subprocess.run()” method to capture the output of the command. You can then access the output as a string using the “result.stdout” attribute:
output = result.stdout.decode('utf-8')
The “decode()” method is used to convert the output from bytes to a string.
Finally, you can parse the output to extract the information you need. For example, to get the total disk space, you can split the output into lines and extract the second line:
lines = output.split('\n')
total_space = lines[1].split()[1]
This will give you the total disk space in bytes. You can convert it to a more readable format using the “humanize” module:
import humanize
total_space_readable = humanize.naturalsize(int(total_space))
And that’s it! With just a few lines of code, you can use the “df” command in Python to check disk space usage on your Linux system.
Equivalent of linux df in python
In conclusion, the `psutil` module in Python provides an easy and efficient way to retrieve disk usage information, similar to the `df` command in Linux. With just a few lines of code, you can access detailed information about disk usage, including total, used, and free space. Additionally, the `psutil` module offers a range of other system monitoring capabilities, making it a valuable tool for any Python developer. Whether you’re working on a personal project or a large-scale application, the `psutil` module is a powerful resource that can help you optimize your code and improve system performance. So, if you’re looking for a reliable and efficient way to monitor disk usage in Python, be sure to check out the `psutil` module.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |