The Linux kill command is used to terminate a running process by sending a signal to it. The signal can be specified by its signal number or name, and the process can be identified by its process ID (PID) or by its name. The default signal sent by the kill command is SIGTERM, which allows the process to perform cleanup operations before terminating. However, if the process does not respond to SIGTERM, a more forceful signal such as SIGKILL can be sent to terminate it immediately. The kill command is a powerful tool that should be used with caution, as terminating a process abruptly can cause data loss or other unintended consequences.. Keep reading below to learn how to linux kill in python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
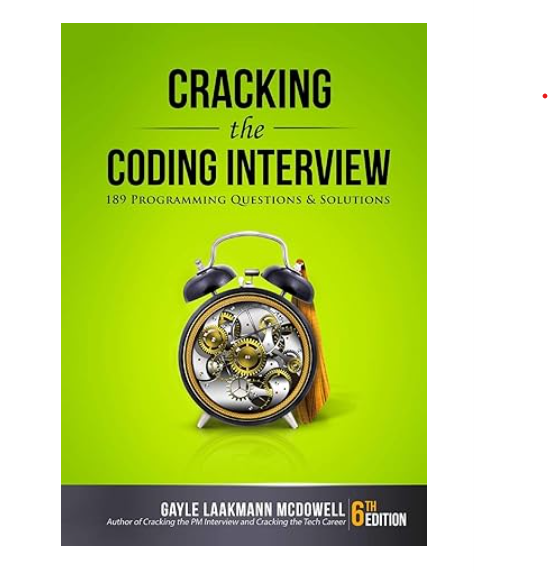
Linux ‘kill’ in Python With Example Code
When working with Linux, it is common to need to terminate a process that is running. This can be done using the kill
command in the terminal. However, if you are working with Python, you may want to terminate a process programmatically. In this blog post, we will explore how to use Python to kill a process in Linux.
The first step is to import the os
module, which provides a way to interact with the operating system. We will also need to import the signal
module, which provides a way to send signals to processes.
Next, we need to identify the process that we want to kill. We can do this using the psutil
module, which provides an interface for retrieving information about running processes. We can use the pid_exists()
function to check if a process with a given PID exists, and the Process()
function to create a Process
object for a given PID.
Once we have identified the process that we want to kill, we can send a signal to it using the kill()
function from the os
module. We can specify the signal that we want to send using the constants defined in the signal
module. For example, to send a SIGTERM signal, we would use the signal.SIGTERM
constant.
Here is an example of how to kill a process with a given PID:
import os
import signal
import psutil
pid = 1234
if psutil.pid_exists(pid):
process = psutil.Process(pid)
os.kill(pid, signal.SIGTERM)
print(f"Process {pid} has been terminated.")
else:
print(f"No process with PID {pid} exists.")
In this example, we first check if a process with the given PID exists using the pid_exists()
function. If it does, we create a Process
object for the process using the Process()
function. We then send a SIGTERM signal to the process using the kill()
function. Finally, we print a message indicating that the process has been terminated.
Using Python to kill processes in Linux can be a useful tool for automating tasks or managing system resources. By combining the os
, signal
, and psutil
modules, we can easily identify and terminate processes programmatically.
Equivalent of linux kill in python
In conclusion, the equivalent of the Linux kill command in Python is the os.kill() function. This function allows you to send a signal to a process, just like the kill command in Linux. With the os.kill() function, you can terminate a process, stop it, or even restart it. This function is a powerful tool for managing processes in Python, and it can be used in a variety of applications. Whether you are working on a small project or a large-scale application, the os.kill() function is a valuable tool to have in your arsenal. So, if you are looking for a way to manage processes in Python, be sure to check out the os.kill() function.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |