The Linux ps command is used to display information about the currently running processes on a system. It provides a snapshot of the system’s process table, including the process ID (PID), the user who started the process, the CPU and memory usage, and the command that started the process. The ps command can be used with various options to filter and sort the output, making it a powerful tool for monitoring and managing processes on a Linux system.. Keep reading below to learn how to linux ps in python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
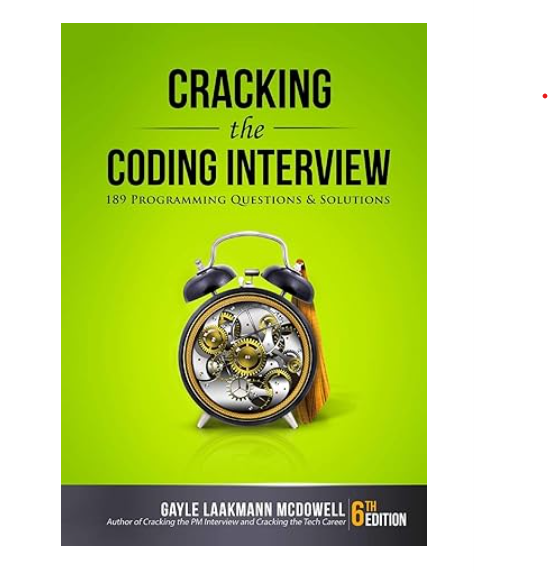
Linux ‘ps’ in Python With Example Code
Linux ps is a command that displays information about running processes on a Linux system. In this blog post, we will learn how to use ps in Python.
To use ps in Python, we will use the subprocess module. The subprocess module allows us to spawn new processes, connect to their input/output/error pipes, and obtain their return codes.
First, we need to import the subprocess module:
import subprocess
Next, we can use the subprocess.run() function to run the ps command and capture its output:
output = subprocess.run(['ps', '-aux'], capture_output=True, text=True)
In this example, we are running the ps command with the -aux options, which displays information about all running processes. We are also using the capture_output=True and text=True options to capture the output as a string.
We can then print the output to the console:
print(output.stdout)
This will print the output of the ps command to the console.
We can also use the output of the ps command in our Python program. For example, we can split the output into lines and extract information about specific processes:
lines = output.stdout.split('\n')
for line in lines:
if 'python' in line:
print(line)
In this example, we are splitting the output into lines and iterating over each line. We are then checking if the line contains the word "python" and printing it to the console if it does.
In conclusion, using ps in Python is a powerful way to obtain information about running processes on a Linux system. By using the subprocess module, we can easily capture the output of the ps command and use it in our Python programs.
Equivalent of linux ps in python
In conclusion, the psutil library in Python provides a powerful and efficient way to retrieve information about running processes on a system. With its easy-to-use functions, developers can quickly and easily access information such as process IDs, CPU usage, memory usage, and more. The equivalent of the Linux ps command in Python can be achieved using the psutil library, making it a valuable tool for system administrators and developers alike. Whether you're monitoring system performance or building a custom process management tool, psutil is a must-have library for any Python developer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |