The Linux scp command is used to securely transfer files between a local and a remote host over a network. It uses the SSH protocol to encrypt the data being transferred, ensuring that it is secure and cannot be intercepted by unauthorized parties. The command syntax is similar to that of the cp command, with the addition of the remote host and destination directory. It can be used to transfer individual files or entire directories, and supports various options such as recursive copying, preserving file permissions, and compressing data during transfer.. Keep reading below to learn how to linux scp in python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
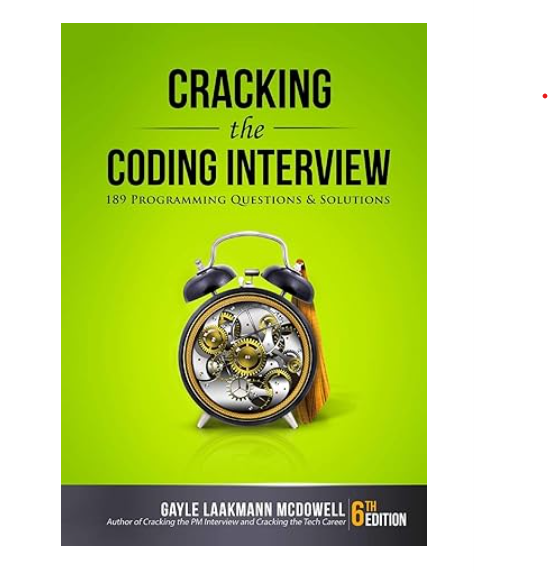
Linux ‘scp’ in Python With Example Code
SCP (Secure Copy) is a protocol used to securely transfer files between remote hosts. In this tutorial, we will learn how to use SCP in Python to transfer files between two Linux machines.
First, we need to install the paramiko
module, which provides an implementation of the SSHv2 protocol. We can install it using pip:
pip install paramiko
Once we have installed the paramiko
module, we can use it to create an SSH connection to the remote host and transfer files using SCP.
Here is an example code snippet that demonstrates how to transfer a file from a remote host to the local machine:
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('remote_host', username='username', password='password')
sftp = ssh.open_sftp()
sftp.get('/remote/path/to/file', '/local/path/to/file')
sftp.close()
ssh.close()
In the above code, we first create an SSH connection to the remote host using the paramiko.SSHClient()
class. We then set the missing host key policy to paramiko.AutoAddPolicy()
to automatically add the host key to the local ~/.ssh/known_hosts
file if it is not already present. We then connect to the remote host using the connect()
method, passing in the hostname, username, and password.
Next, we open an SFTP session using the open_sftp()
method and use the get()
method to transfer the file from the remote host to the local machine. Finally, we close the SFTP session and the SSH connection.
We can also transfer files from the local machine to the remote host using SCP. Here is an example code snippet that demonstrates how to transfer a file from the local machine to the remote host:
import paramiko
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect('remote_host', username='username', password='password')
sftp = ssh.open_sftp()
sftp.put('/local/path/to/file', '/remote/path/to/file')
sftp.close()
ssh.close()
In the above code, we use the put()
method to transfer the file from the local machine to the remote host.
That’s it! We have learned how to use SCP in Python to transfer files between two Linux machines.
Equivalent of linux scp in python
In conclusion, the equivalent of the Linux scp command in Python is the paramiko module. This module provides a secure way to transfer files between remote servers using the SSH protocol. With its easy-to-use API, developers can easily integrate file transfer functionality into their Python applications. The paramiko module also offers additional features such as remote command execution and shell access, making it a versatile tool for managing remote servers. Overall, the paramiko module is a reliable and efficient solution for transferring files securely between remote servers using Python.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |