The Linux touch command is used to create a new empty file or update the timestamp of an existing file. When used with a filename, touch will create a new file with that name if it does not already exist. If the file already exists, touch will update the timestamp of the file to the current time. Touch can also be used to update the timestamp of multiple files at once by specifying multiple filenames as arguments. Additionally, touch can be used to create a file with a specific timestamp by specifying the desired timestamp using the -t option.. Keep reading below to learn how to linux touch in python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
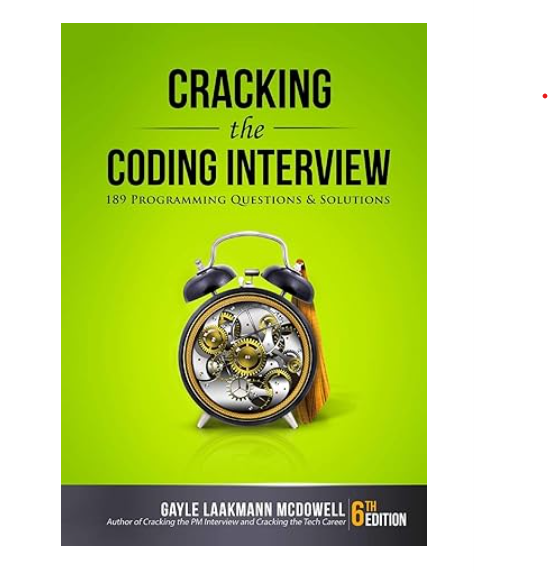
Linux ‘touch’ in Python With Example Code
Linux touch command is used to create a new empty file or update the modification and access times of an existing file. In Python, we can use the os
module to execute the touch command.
To create a new file using touch in Python, we can use the os.mknod()
method. This method creates a new file with the specified name and mode. Here’s an example:
import os
os.mknod("newfile.txt")
This will create a new file named newfile.txt
in the current directory.
If you want to update the modification and access times of an existing file, you can use the os.utime()
method. This method takes two arguments: the path of the file and a tuple containing the new access and modification times. Here’s an example:
import os
import time
# get the current time
now = time.time()
# update the modification and access times of the file
os.utime("existingfile.txt", (now, now))
This will update the modification and access times of the file named existingfile.txt
to the current time.
Equivalent of linux touch in python
In conclusion, the touch command in Linux is a powerful tool for creating and modifying files. However, Python provides an equivalent functionality through the os module. By using the os module’s `open()` function, we can create and modify files in Python. Additionally, the `os.utime()` function can be used to modify the access and modification times of a file. With these tools, Python developers can easily manipulate files and directories in their programs. Whether you are a Linux user or a Python developer, understanding the touch command and its Python equivalent can be a valuable skill to have.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |