The Linux zip command is used to compress and archive files and directories into a single file with the .zip extension. It allows users to compress files and directories to save disk space, transfer files more efficiently, and create backups. The command can be used to add, update, and delete files from an existing zip archive, as well as to encrypt and password-protect the archive. The zip command is commonly used in Linux systems for file compression and archiving purposes.. Keep reading below to learn how to linux zip in python.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
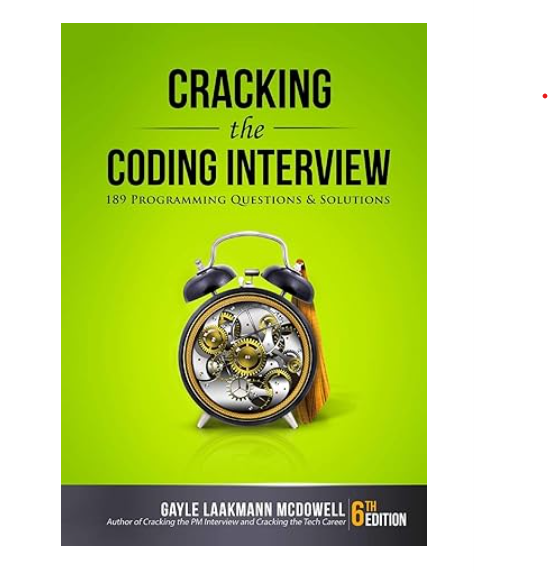
Linux ‘zip’ in Python With Example Code
Zip files are a common way to compress and package files in Linux. Python provides a built-in module called zipfile
that allows you to create, read, and extract zip files. In this tutorial, we will learn how to use the zipfile
module in Python to zip files and directories.
To get started, we need to import the zipfile
module:
import zipfile
Next, we can create a new zip file using the ZipFile()
method:
with zipfile.ZipFile('example.zip', 'w') as zip:
zip.write('file.txt')
zip.write('folder')
This will create a new zip file called example.zip
and add the file.txt
and folder
to it. The write()
method is used to add files and directories to the zip file.
If you want to add files from a specific directory, you can use the walk()
method to iterate over all the files in the directory:
import os
with zipfile.ZipFile('example.zip', 'w') as zip:
for foldername, subfolders, filenames in os.walk('myfolder'):
for filename in filenames:
filepath = os.path.join(foldername, filename)
zip.write(filepath)
This will add all the files in the myfolder
directory and its subdirectories to the example.zip
file.
To extract files from a zip file, we can use the extractall()
method:
with zipfile.ZipFile('example.zip', 'r') as zip:
zip.extractall('extracted')
This will extract all the files in the example.zip
file to a new directory called extracted
.
That’s it! You now know how to use the zipfile
module in Python to zip and extract files and directories.
Equivalent of linux zip in python
In conclusion, the equivalent of the Linux zip command in Python is the zipfile module. This module provides a simple and efficient way to create, read, and extract zip archives in Python. With the zipfile module, you can easily compress and decompress files and directories, and even password-protect your archives. Additionally, the module offers a range of options and methods to customize your zip archives, making it a versatile tool for managing your files. Whether you’re a beginner or an experienced Python developer, the zipfile module is a valuable addition to your toolkit. So, next time you need to zip or unzip files in Python, give the zipfile module a try!
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |