The abs() function in Python returns the absolute value of a number. The absolute value of a number is its distance from zero on the number line, regardless of whether the number is positive or negative. The abs() function takes a single argument, which can be an integer, a floating-point number, or a complex number. If the argument is an integer or a floating-point number, the function returns the absolute value of that number. If the argument is a complex number, the function returns the magnitude of that number, which is the distance from the origin to the point representing the complex number in the complex plane. The abs() function is a built-in function in Python, so it can be used without importing any modules.. Keep reading below to learn how to python abs in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
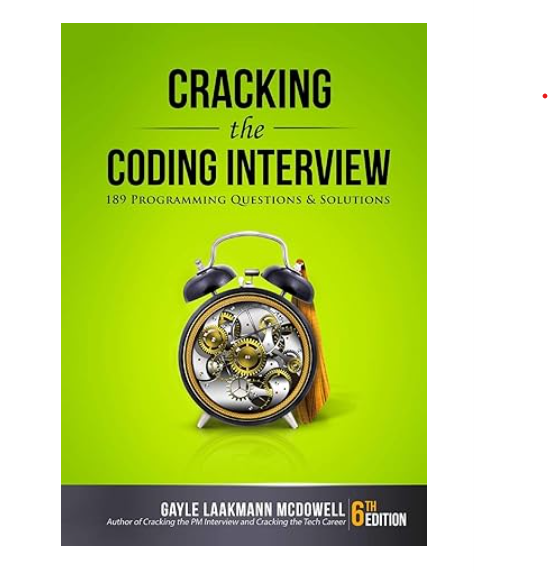
Python ‘abs’ in C++ With Example Code
Python’s built-in `abs()` function returns the absolute value of a number. In C++, there is no built-in function for absolute value, but it can be easily implemented using the ternary operator.
To implement `abs()` in C++, we can use the ternary operator to check if the number is negative. If it is negative, we return the negation of the number, otherwise we return the number itself.
Here’s the code:
“`cpp
int abs(int num) {
return (num < 0) ? -num : num;
}
```
In this code, we define a function called `abs` that takes an integer `num` as its argument. We then use the ternary operator to check if `num` is less than 0. If it is, we return the negation of `num` (i.e. `-num`), otherwise we return `num` itself.
We can then use this function to get the absolute value of any integer in our C++ program.
```cpp
int main() {
int num = -5;
int abs_num = abs(num);
cout << "The absolute value of " << num << " is " << abs_num << endl;
return 0;
}
```
In this example, we define an integer `num` with a value of -5. We then call our `abs` function to get the absolute value of `num`, and store the result in a new integer called `abs_num`. Finally, we print out the original value of `num` and its absolute value using `cout`.
And that's it! With this simple implementation, we can easily get the absolute value of any integer in C++.
Equivalent of Python abs in C++
In conclusion, the equivalent function of Python’s abs() in C++ is the abs() function from the
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |