The abs() function in Python returns the absolute value of a number. The absolute value of a number is its distance from zero on the number line, regardless of whether the number is positive or negative. The abs() function takes a single argument, which can be an integer, a floating-point number, or a complex number. If the argument is an integer or a floating-point number, the function returns the absolute value of that number. If the argument is a complex number, the function returns the magnitude of that number, which is the distance from the origin to the point representing the complex number in the complex plane. The abs() function is a built-in function in Python, so it can be used without importing any modules. Keep reading below to learn how to python abs in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
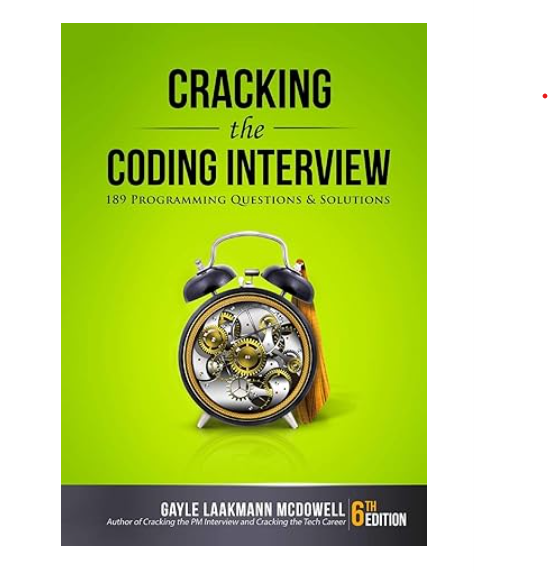
Python ‘abs’ in Go With Example Code
Python’s built-in `abs()` function returns the absolute value of a number. In Go, there is no built-in function for absolute value, but it can be easily implemented using a simple if statement.
To implement absolute value in Go, we can create a function that takes a number as an argument and returns its absolute value. Here’s an example:
func abs(n int) int {
if n < 0 {
return -n
}
return n
}
In this function, we check if the number is less than 0. If it is, we return the negative of the number. Otherwise, we return the number itself.
To use this function, simply call it with a number as an argument:
fmt.Println(abs(-5)) // Output: 5
fmt.Println(abs(5)) // Output: 5
In the first example, we pass -5 to the `abs()` function, which returns 5. In the second example, we pass 5 to the function, which also returns 5.
Implementing absolute value in Go is simple and straightforward, and can be done with just a few lines of code.
Equivalent of Python abs in Go
In conclusion, the equivalent of the Python abs() function in Go is the math.Abs() function. This function takes a float64 value as its argument and returns its absolute value. It is a simple and efficient way to calculate the absolute value of a number in Go. Whether you are a beginner or an experienced Go developer, understanding the math.Abs() function is essential for writing clean and effective code. So, if you are looking for a way to calculate the absolute value of a number in Go, the math.Abs() function is the way to go!
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |