The abs() function in Python returns the absolute value of a number. The absolute value of a number is its distance from zero on the number line, regardless of whether the number is positive or negative. The abs() function takes a single argument, which can be an integer, a floating-point number, or a complex number. If the argument is an integer or a floating-point number, the function returns the absolute value of that number. If the argument is a complex number, the function returns the magnitude of that number, which is the distance from the origin to the point representing the complex number in the complex plane. The abs() function is a built-in function in Python, so it can be used without importing any modules. Keep reading below to learn how to python abs in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
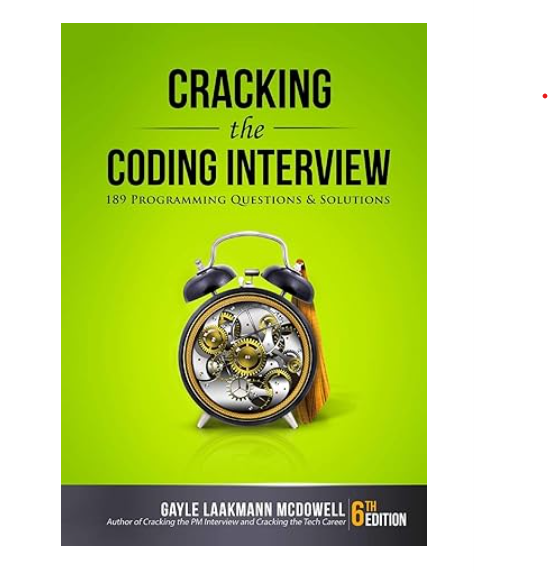
Python ‘abs’ in Javascript With Example Code
If you’re familiar with Python, you may have used the abs()
function to return the absolute value of a number. In JavaScript, there is no built-in abs()
function, but it’s easy to create one using a conditional statement.
Here’s an example:
function abs(num) {
if (num < 0) {
return -num;
} else {
return num;
}
}
This function takes a number as an argument and returns its absolute value. If the number is less than 0, it returns the negative of the number. Otherwise, it returns the number itself.
You can use this function just like you would use the abs()
function in Python:
console.log(abs(-5)); // Output: 5
console.log(abs(5)); // Output: 5
With this simple function, you can easily find the absolute value of any number in JavaScript.
Equivalent of Python abs in Javascript
In conclusion, the equivalent function of Python’s abs() in JavaScript is Math.abs(). Both functions serve the same purpose of returning the absolute value of a number, regardless of its sign. It is important to note that while the syntax and implementation may differ between the two languages, the concept remains the same. As a developer, it is crucial to have a good understanding of the various programming languages and their functions to be able to write efficient and effective code. With this knowledge, you can easily switch between languages and use the appropriate functions to achieve your desired results.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |