The abs() function in Python returns the absolute value of a number. The absolute value of a number is its distance from zero on the number line, regardless of whether the number is positive or negative. The abs() function takes a single argument, which can be an integer, a floating-point number, or a complex number. If the argument is an integer or a floating-point number, the function returns the absolute value of that number. If the argument is a complex number, the function returns the magnitude of that number, which is the distance from the origin to the point representing the complex number in the complex plane. The abs() function is a built-in function in Python, so it can be used without importing any modules. Keep reading below to learn how to python abs in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
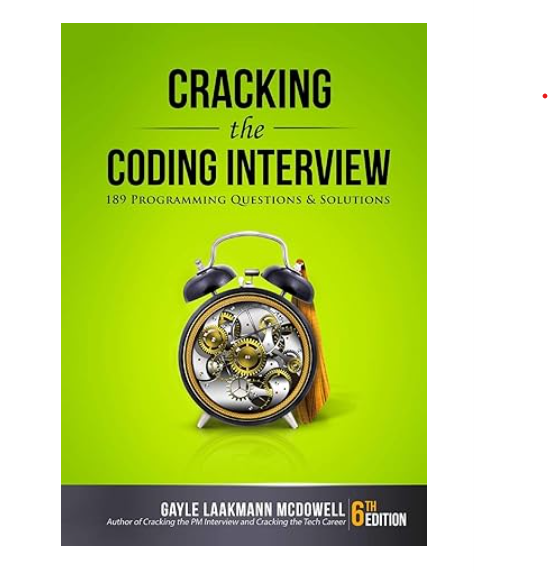
Python ‘abs’ in Rust With Example Code
Python’s built-in `abs()` function returns the absolute value of a number. Rust, being a systems programming language, does not have a built-in `abs()` function. However, it is easy to implement one using Rust’s standard library.
To implement `abs()` in Rust, we can use the `num::abs()` function from the `num` crate. This function returns the absolute value of a number, and works with any numeric type that implements the `Signed` trait.
Here’s an example implementation of `abs()` in Rust:
use num::abs;
fn abs(n: i32) -> i32 {
abs(n)
}
In this example, we import the `abs()` function from the `num` crate, and define our own `abs()` function that simply calls the `num::abs()` function with the given argument.
To use this function in your Rust code, simply call it with a numeric value:
let n = -5;
let abs_n = abs(n);
println!("The absolute value of {} is {}", n, abs_n);
This will output:
The absolute value of -5 is 5
With this implementation, you can easily use `abs()` in your Rust code, just like you would in Python.
Equivalent of Python abs in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to perform mathematical operations, including finding the absolute value of a number. The equivalent of the Python abs() function in Rust is the abs() method, which can be used on any numeric data type. This method returns the absolute value of the input number, regardless of whether it is positive or negative. With Rust’s focus on performance and safety, the abs() method is a reliable and efficient way to perform this common mathematical operation in your Rust programs. Whether you are a beginner or an experienced Rust developer, the abs() method is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |