The abs() function in Python returns the absolute value of a number. The absolute value of a number is its distance from zero on the number line, regardless of whether the number is positive or negative. The abs() function takes a single argument, which can be an integer, a floating-point number, or a complex number. If the argument is an integer or a floating-point number, the function returns the absolute value of that number. If the argument is a complex number, the function returns the magnitude of that number, which is the distance from the origin to the point representing the complex number in the complex plane. The abs() function is a built-in function in Python, so it can be used without importing any modules. Keep reading below to learn how to python abs in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
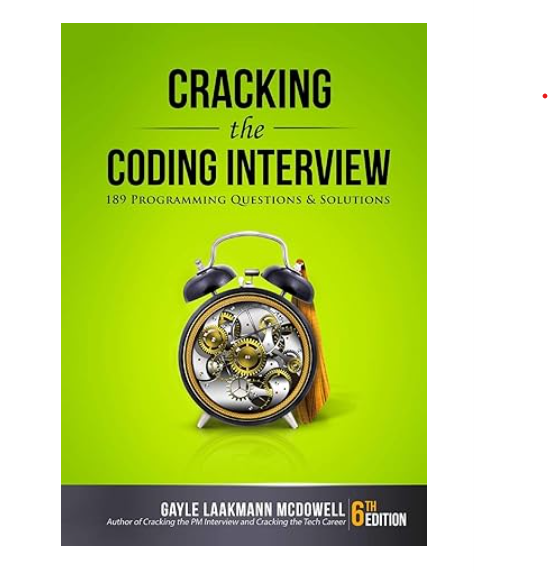
Python ‘abs’ in TypeScript With Example Code
Python’s built-in `abs()` function returns the absolute value of a number. TypeScript, being a superset of JavaScript, does not have a built-in `abs()` function for numbers. However, we can easily create our own implementation of `abs()` in TypeScript.
To create a TypeScript implementation of `abs()`, we can use the following code:
function abs(num: number): number {
return num < 0 ? -num : num;
}
In this code, we define a function called `abs` that takes a single parameter `num` of type `number`. The function then checks if `num` is less than 0. If it is, the function returns the negation of `num` (i.e., `-num`), which is the absolute value of `num`. If `num` is greater than or equal to 0, the function simply returns `num`.
We can then use this `abs()` function in our TypeScript code to get the absolute value of a number. For example:
const num = -10;
const absNum = abs(num);
console.log(absNum); // Output: 10
In this code, we define a variable `num` and assign it the value -10. We then call our `abs()` function with `num` as the argument and assign the result to a variable called `absNum`. Finally, we log the value of `absNum` to the console, which should output 10.
With this implementation of `abs()` in TypeScript, we can easily get the absolute value of any number in our code.
Equivalent of Python abs in TypeScript
In conclusion, TypeScript provides a built-in function called `Math.abs()` that is equivalent to the Python `abs()` function. Both functions return the absolute value of a number, regardless of its sign. The `Math.abs()` function can be used in TypeScript to perform mathematical operations and calculations that require the absolute value of a number. It is important to note that TypeScript is a strongly-typed language, which means that the type of the input parameter must be specified when using the `Math.abs()` function. Overall, the `Math.abs()` function in TypeScript is a useful tool for developers who need to perform mathematical operations and calculations in their TypeScript code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |