The Python any() function is a built-in function that returns True if at least one element in an iterable object is true, and False if all elements are false or the iterable is empty. It takes an iterable object as an argument and checks each element in the iterable for truthiness. If any element is true, the function returns True. If all elements are false or the iterable is empty, the function returns False. The any() function is commonly used in conditional statements and loops to check if any element in a list, tuple, or other iterable object meets a certain condition.. Keep reading below to learn how to python any in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
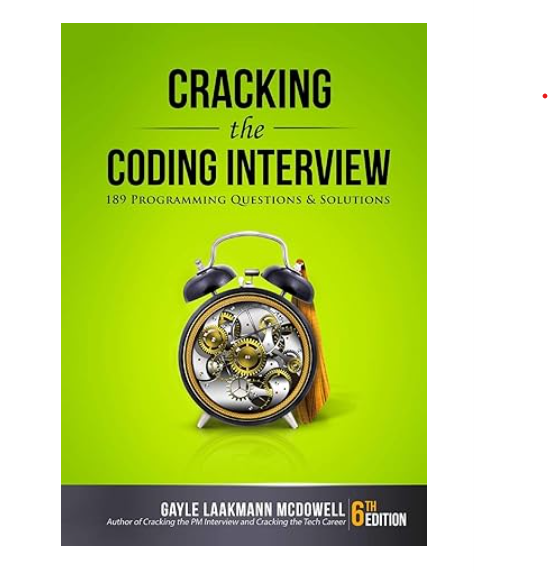
Python ‘any’ in C# With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. However, there may be times when you need to use C# instead. In this blog post, we will show you how to use Python’s “any” function in C#.
The “any” function in Python is used to determine if any element in an iterable is true. In C#, you can achieve the same functionality using the “Enumerable.Any” method. This method takes an IEnumerable
To use the “Enumerable.Any” method, you first need to import the System.Linq namespace. This namespace contains the Enumerable class, which provides a set of extension methods for working with collections.
Once you have imported the System.Linq namespace, you can use the “Enumerable.Any” method in your code. Here is an example:
“`
using System.Linq;
class Program
{
static void Main(string[] args)
{
int[] numbers = { 1, 2, 3, 4, 5 };
bool anyGreaterThanThree = numbers.Any(n => n > 3);
Console.WriteLine(anyGreaterThanThree);
}
}
“`
In this example, we have an array of integers called “numbers”. We use the “Enumerable.Any” method to determine if any element in the array is greater than three. The lambda expression “n => n > 3” is used to specify the condition that we want to check.
The output of this program will be “True”, since there are elements in the array that are greater than three.
In conclusion, while Python and C# are different programming languages, they share many similarities. By using the “Enumerable.Any” method in C#, you can achieve the same functionality as Python’s “any” function.
Equivalent of Python any in C#
In conclusion, the equivalent of the Python any() function in C# is the Enumerable.Any() method. This method allows you to check if any element in a collection satisfies a given condition. It is a powerful tool that can be used to simplify your code and make it more efficient. By using the Enumerable.Any() method, you can easily determine if a collection contains any elements that meet your criteria, without having to write complex loops or conditional statements. Whether you are a beginner or an experienced developer, the Enumerable.Any() method is a valuable tool to have in your programming arsenal. So, if you are looking for a way to simplify your code and make it more efficient, be sure to give the Enumerable.Any() method a try in your next C# project.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |