The Python any() function is a built-in function that returns True if at least one element in an iterable object is true, and False if all elements are false or the iterable is empty. It takes an iterable object as an argument and checks each element in the iterable for truthiness. If any element is true, the function returns True. If all elements are false or the iterable is empty, the function returns False. The any() function is commonly used in conditional statements and loops to check if any element in a list, tuple, or other iterable object meets a certain condition. Keep reading below to learn how to python any in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
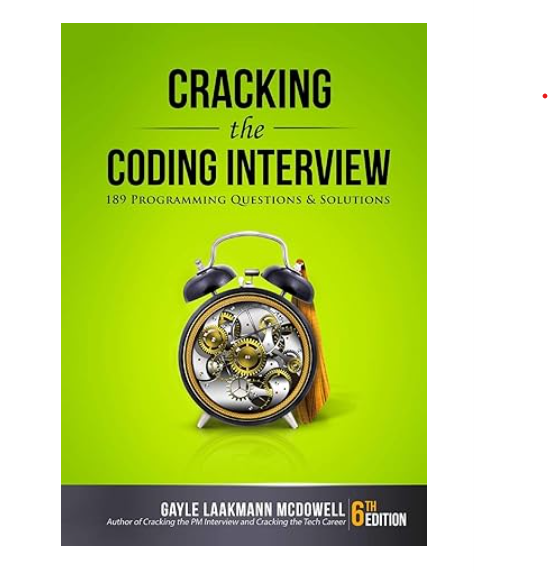
Python ‘any’ in Go With Example Code
Python’s `any` function is a useful tool for checking if any element in an iterable is true. Go, being a statically typed language, does not have a built-in equivalent to Python’s `any`. However, we can easily create our own implementation.
To create a `any` function in Go, we can define a function that takes in an iterable and a function that returns a boolean. The function will iterate over the iterable and apply the function to each element. If any element returns true, the function will return true. Otherwise, it will return false.
Here’s an example implementation:
func any(iterable []interface{}, f func(interface{}) bool) bool {
for _, item := range iterable {
if f(item) {
return true
}
}
return false
}
In this implementation, we define a function called `any` that takes in an iterable of type `[]interface{}` and a function that takes in an interface and returns a boolean. We then iterate over the iterable using a `for` loop and apply the function to each element using `f(item)`. If any element returns true, we immediately return true. If we iterate over the entire iterable and no element returns true, we return false.
We can use this function to check if any element in an iterable is true. For example, let’s say we have a slice of integers:
numbers := []int{1, 2, 3, 4, 5}
We can use our `any` function to check if any element in this slice is even:
result := any(numbers, func(i interface{}) bool {
return i.(int)%2 == 0
})
fmt.Println(result) // Output: true
In this example, we pass in our `numbers` slice and a function that checks if an integer is even. Our `any` function returns true because there is at least one even number in the slice.
With this implementation, we can easily create our own `any` function in Go and use it to check if any element in an iterable is true.
Equivalent of Python any in Go
In conclusion, the equivalent of the Python `any()` function in Go is the `contains()` function from the `strings` package. While the syntax and usage may differ slightly between the two languages, both functions serve the same purpose of checking if any element in a collection meets a certain condition. It’s important to note that the `contains()` function only works with strings, so if you need to check for the presence of a specific element in a different type of collection, you may need to use a different function or approach. Overall, understanding the similarities and differences between these functions in different programming languages can help you become a more versatile and effective developer.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |