The Python any() function is a built-in function that returns True if at least one element in an iterable object is true, and False if all elements are false or the iterable is empty. It takes an iterable object as an argument and checks each element in the iterable for truthiness. If any element is true, the function returns True. If all elements are false or the iterable is empty, the function returns False. The any() function is commonly used in conditional statements and loops to check if any element in a list, tuple, or other iterable object meets a certain condition.. Keep reading below to learn how to python any in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
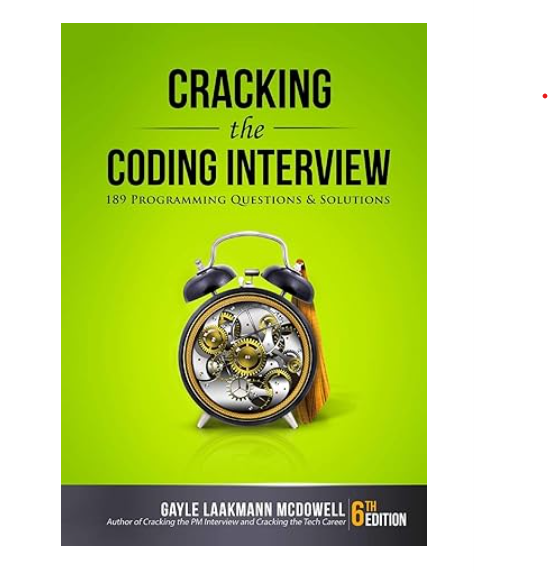
Python ‘any’ in Java With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. However, there may be times when you need to use Java instead. In this blog post, we will discuss how to use the “any” function in Python and its equivalent in Java.
The “any” function in Python is used to determine if any element in an iterable is true. It returns True if at least one element is true, and False otherwise. Here is an example:
“`
my_list = [False, False, True, False]
if any(my_list):
print(“At least one element is true”)
else:
print(“No element is true”)
“`
The output of this code will be “At least one element is true” because the third element in the list is True.
In Java, there is no direct equivalent to the “any” function in Python. However, you can achieve the same result using a for loop. Here is an example:
“`
boolean[] myArray = {false, false, true, false};
boolean anyTrue = false;
for (boolean b : myArray) {
if (b) {
anyTrue = true;
break;
}
}
if (anyTrue) {
System.out.println(“At least one element is true”);
} else {
System.out.println(“No element is true”);
}
“`
The output of this code will be the same as the Python code: “At least one element is true”.
In conclusion, while there is no direct equivalent to the “any” function in Java, you can achieve the same result using a for loop.
Equivalent of Python any in Java
In conclusion, while Python’s `any()` function is a convenient way to check if any element in an iterable is true, Java does not have an equivalent built-in function. However, we can easily implement the same functionality using a loop and a boolean flag. By iterating through the collection and checking each element, we can set the flag to true if any element is true. This approach allows us to achieve the same result as the `any()` function in Python. While it may require a bit more code, it is a simple and effective solution that can be used in any Java project.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |