The Python any() function is a built-in function that returns True if at least one element in an iterable object is true, and False if all elements are false or the iterable is empty. It takes an iterable object as an argument and checks each element in the iterable for truthiness. If any element is true, the function returns True. If all elements are false or the iterable is empty, the function returns False. The any() function is commonly used in conditional statements and loops to check if any element in a list, tuple, or other iterable object meets a certain condition. Keep reading below to learn how to python any in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
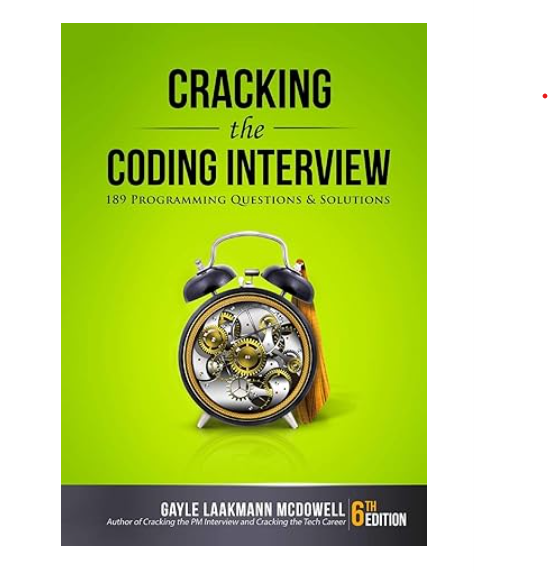
Python ‘any’ in Kotlin With Example Code
Python’s `any` function is a useful tool for checking if at least one element in an iterable is true. Kotlin doesn’t have a built-in `any` function, but it’s easy to create one using higher-order functions.
To create an `any` function in Kotlin, we can use the `fold` higher-order function. `fold` takes an initial value and a lambda function that takes two arguments: the accumulator and the current element. The lambda function returns the new accumulator value.
Here’s an example implementation of `any` in Kotlin:
fun
return this.fold(false) { acc, element -> acc || predicate(element) }
}
In this implementation, we start with an initial value of `false` for the accumulator. We then iterate over each element in the iterable and apply the `predicate` function to it. If the `predicate` function returns `true` for any element, we set the accumulator to `true` and return it.
To use this `myAny` function, we can call it on any iterable and pass in a lambda function that takes an element and returns a boolean value. Here’s an example usage:
val numbers = listOf(1, 2, 3, 4, 5)
val hasEvenNumber = numbers.myAny { it % 2 == 0 }
println(hasEvenNumber) // true
In this example, we’re checking if the `numbers` list contains any even numbers. The lambda function `{ it % 2 == 0 }` returns `true` if the element is even, and `false` otherwise. The `myAny` function returns `true` because at least one element in the list is even.
With this implementation of `any`, we can easily check if any element in an iterable satisfies a condition, just like we can with Python’s `any` function.
Equivalent of Python any in Kotlin
In conclusion, the equivalent of the Python `any()` function in Kotlin is the `any()` extension function. This function can be used to check if at least one element in a collection satisfies a given condition. It is a useful tool for simplifying code and improving readability. By using the `any()` function, Kotlin developers can easily check for the presence of a specific element in a collection without having to write complex loops or conditionals. Overall, the `any()` function is a powerful feature of Kotlin that can help developers write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |