The Python ascii() function returns a string containing a printable representation of an object. It takes an object as an argument and returns a string that represents the object in ASCII encoding. The function replaces non-ASCII characters with escape sequences, such as \x, \u, or \U, followed by the hexadecimal representation of the character code. The ascii() function is useful for debugging and displaying non-printable characters in a readable format. It can be used with strings, numbers, lists, tuples, dictionaries, and other objects. Keep reading below to learn how to python ascii in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
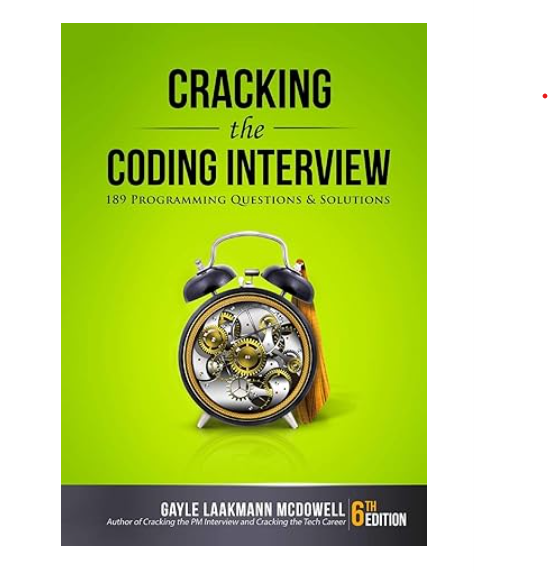
Python ‘ascii’ in Kotlin With Example Code
Python ASCII art is a fun way to add some visual interest to your code. But did you know that you can also create ASCII art in Kotlin? In this post, we’ll show you how to create ASCII art in Kotlin using Python’s ASCII art library.
To get started, you’ll need to install the Python library `art` using pip. Open up your terminal and type:
pip install art
Once you have the library installed, you can start creating ASCII art in Kotlin. Here’s an example:
import com.github.ajalt.mordant.TermColors
import com.github.ajalt.mordant.rendering.TextColors
import com.github.ajalt.mordant.terminal.Terminal
fun main() {
val t = Terminal()
val colors = TermColors(TermColors.Level.TRUECOLOR)
val text = "Hello, world!"
val asciiArt = colors.asciiArt(text) {
font = "block"
color = TextColors.random()
}
t.println(asciiArt)
}
In this example, we’re using the `art` library to create ASCII art from the text “Hello, world!”. We’re also using the `mordant` library to add some color to our ASCII art.
To create the ASCII art, we’re using the `asciiArt` function from the `TermColors` class. We’re passing in the text we want to create the art from, and then using the `font` and `color` properties to customize the look of the art.
Finally, we’re using the `Terminal` class to print out the ASCII art to the console.
And that’s it! With just a few lines of code, you can create your own ASCII art in Kotlin. Give it a try and see what kind of cool designs you can come up with!
Equivalent of Python ascii in Kotlin
In conclusion, the equivalent function of Python’s `ascii()` in Kotlin is the `toByte()` function. Both functions serve the purpose of converting a given character or string into its corresponding ASCII code. However, it is important to note that the `toByte()` function in Kotlin returns the ASCII code as a byte value, whereas the `ascii()` function in Python returns the ASCII code as a string. Overall, Kotlin provides a simple and efficient way to convert characters and strings into their ASCII codes using the `toByte()` function. This function can be used in various applications, such as encoding and decoding messages, working with binary data, and more. As Kotlin continues to gain popularity among developers, it is important to understand the various functions and features it offers, including the `toByte()` function for ASCII conversion.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |