The Python bin() function is used to convert an integer number to its binary representation. It takes an integer as an argument and returns a string representing the binary equivalent of the input integer. The returned string starts with the prefix ‘0b’ to indicate that it is a binary number. The bin() function is commonly used in computer science and programming to manipulate binary data and perform bitwise operations. It is a built-in function in Python and is easy to use, making it a popular choice for working with binary data.. Keep reading below to learn how to python bin in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
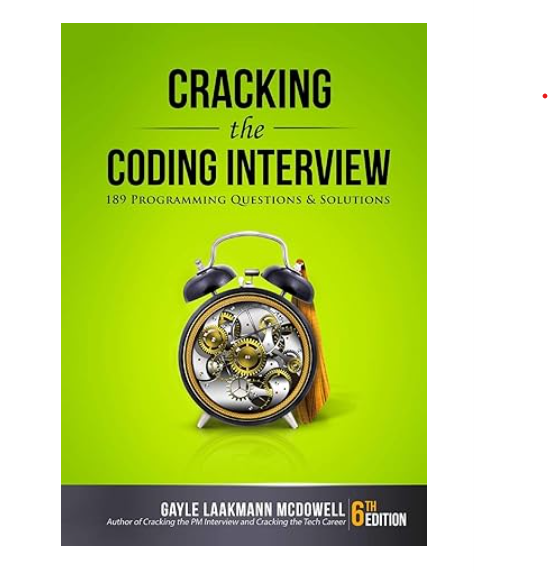
Python ‘bin’ in C# With Example Code
Python’s `bin()` function is a built-in function that returns the binary representation of an integer. In C#, there is no direct equivalent to this function, but it is possible to create a similar function using bitwise operators.
To create a `bin()` function in C#, we can start by creating a method that takes an integer as an argument. We can then use the bitwise AND operator (`&`) to check each bit in the integer and add either a `0` or `1` to a string variable.
“`csharp
public static string Bin(int num)
{
string binary = “”;
for (int i = 31; i >= 0; i–)
{
int bit = num & (1 << i);
binary += bit == 0 ? "0" : "1";
}
return binary;
}
```
In this example, we start at the leftmost bit (bit 31) and work our way to the right (bit 0). We use the bitwise AND operator to check if the bit is a `1` or a `0`. If the bit is a `1`, we add a `1` to the binary string, otherwise we add a `0`.
Once we have checked all 32 bits, we return the binary string.
To use this function, we can simply call it with an integer argument:
```csharp
int num = 42;
string binary = Bin(num);
Console.WriteLine(binary); // Output: 00000000000000000000000000101010
```
This will output the binary representation of the integer `42`.
In conclusion, while C# does not have a built-in `bin()` function like Python, it is possible to create a similar function using bitwise operators.
Equivalent of Python bin in C#
In conclusion, the equivalent function of Python’s bin() in C# is the Convert.ToString() method. This method allows us to convert a decimal number to its binary representation. While the syntax may differ between the two languages, the functionality remains the same. It is important to note that C# offers a wide range of conversion methods for different number systems, including hexadecimal and octal. As a programmer, it is essential to have a good understanding of these conversion methods to efficiently manipulate and process data. By utilizing the Convert.ToString() method, we can easily convert decimal numbers to binary in C# and perform various operations on them.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |