The Python bin() function is used to convert an integer number to its binary representation. It takes an integer as an argument and returns a string representing the binary equivalent of the input integer. The returned string starts with the prefix ‘0b’ to indicate that it is a binary number. The bin() function is commonly used in computer science and programming to manipulate binary data and perform bitwise operations. It is a built-in function in Python and is easy to use, making it a popular choice for working with binary data.. Keep reading below to learn how to python bin in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
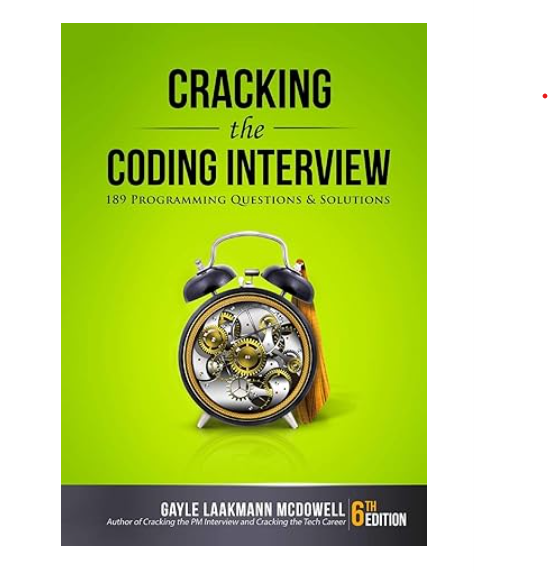
Python ‘bin’ in Java With Example Code
Python’s `bin()` function is used to convert an integer to its binary representation. However, Java does not have a built-in `bin()` function. In this blog post, we will explore how to implement the `bin()` function in Java.
To implement the `bin()` function in Java, we can use the `Integer.toBinaryString()` method. This method takes an integer as an argument and returns its binary representation as a string.
Here’s an example implementation of the `bin()` function in Java:
“`
public static String bin(int n) {
return Integer.toBinaryString(n);
}
“`
We can then use this function to convert an integer to its binary representation:
“`
int num = 10;
String binary = bin(num);
System.out.println(binary); // Output: 1010
“`
In this example, we pass the integer `10` to the `bin()` function, which returns its binary representation as a string. We then print the string to the console.
In conclusion, while Java does not have a built-in `bin()` function like Python, we can easily implement it using the `Integer.toBinaryString()` method.
Equivalent of Python bin in Java
In conclusion, the equivalent function of Python’s bin() in Java is Integer.toBinaryString(). Both functions serve the same purpose of converting a decimal number to its binary representation. However, the syntax and usage of these functions differ slightly. While bin() returns a string with a prefix ‘0b’, toBinaryString() returns a string without any prefix. Additionally, toBinaryString() only works with integer values, whereas bin() can also handle float and complex numbers. Overall, understanding the differences between these functions can help developers choose the appropriate method for their specific use case.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |