The Python bin() function is used to convert an integer number to its binary representation. It takes an integer as an argument and returns a string representing the binary equivalent of the input integer. The returned string starts with the prefix ‘0b’ to indicate that it is a binary number. The bin() function is commonly used in computer science and programming to manipulate binary data, such as in bitwise operations or when working with binary files. It is a built-in function in Python and is easy to use, making it a popular choice for converting integers to binary. Keep reading below to learn how to python bin in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
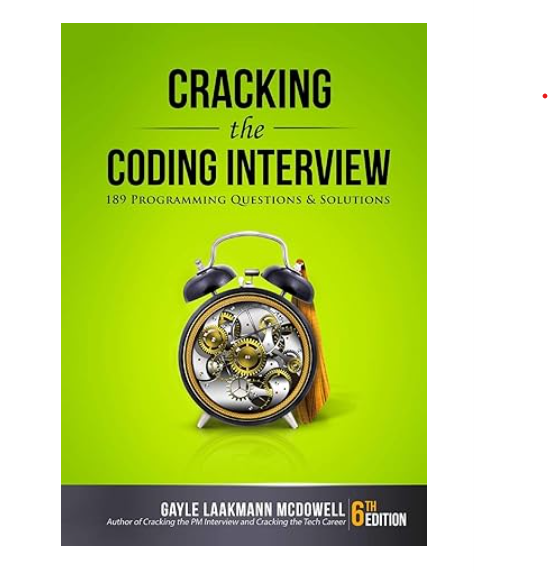
Python ‘bin’ in Javascript With Example Code
Python’s `bin()` function is used to convert an integer to its binary representation. However, JavaScript does not have a built-in equivalent function. In this blog post, we will explore how to implement the `bin()` function in JavaScript.
To start, let’s take a look at the Python `bin()` function:
bin(10)
This would output the binary representation of the integer 10, which is `0b1010`.
To implement this in JavaScript, we can create a function that takes an integer as an argument and returns its binary representation. Here’s an example implementation:
function bin(num) {
return (num >>> 0).toString(2);
}
Let’s break down how this function works. First, we use the bitwise right shift operator (`>>>`) to convert the input number to an unsigned 32-bit integer. This is necessary because JavaScript’s bitwise operators only work on 32-bit integers.
Next, we use the `toString()` method with a base of 2 to convert the unsigned integer to its binary representation.
Now, let’s test our `bin()` function with an example:
console.log(bin(10)); // Output: "1010"
As you can see, our function correctly outputs the binary representation of the input integer.
In conclusion, while JavaScript does not have a built-in `bin()` function like Python, we can easily implement our own using bitwise operators and the `toString()` method.
Equivalent of Python bin in Javascript
In conclusion, the equivalent function of Python’s bin() in JavaScript is the toString() method with a radix of 2. This method converts a number to a string in binary format. While the syntax may differ between the two languages, the functionality remains the same. It is important to note that the toString() method can also be used to convert numbers to other number systems such as octal and hexadecimal by changing the radix parameter. Understanding the equivalent function in different programming languages can help developers to write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |