The Python bin() function is used to convert an integer number to its binary representation. It takes an integer as an argument and returns a string representing the binary equivalent of the input integer. The returned string starts with the prefix ‘0b’ to indicate that it is a binary number. The bin() function is commonly used in computer science and programming to manipulate binary data and perform bitwise operations. It is a built-in function in Python and is easy to use, making it a popular choice for working with binary data. Keep reading below to learn how to python bin in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
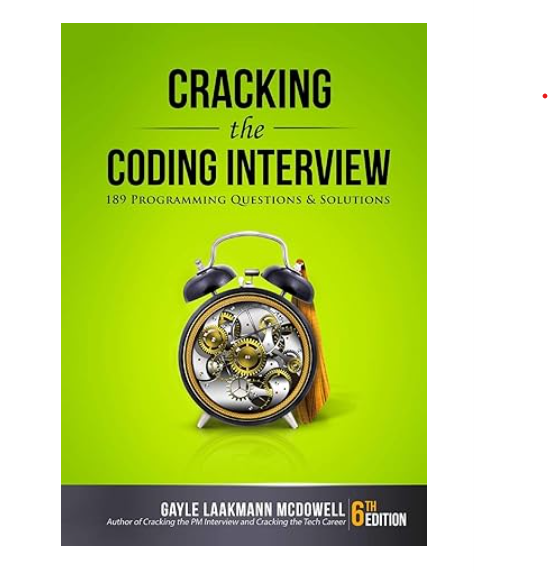
Python ‘bin’ in Rust With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. However, there are times when Python may not be the best choice for a particular task. In such cases, Rust, a systems programming language that is known for its speed and memory safety, can be a great alternative. In this blog post, we will explore how to use Rust’s `std::process::Command` module to execute Python scripts.
To get started, you will need to have Rust installed on your system. You can download Rust from the official website and follow the installation instructions.
Once you have Rust installed, you can create a new Rust project using the following command:
cargo new my_project
This will create a new Rust project in a directory called `my_project`. Next, you will need to add the `std::process::Command` module to your project’s dependencies. You can do this by adding the following line to your `Cargo.toml` file:
[dependencies]
libc = "0.2"
With the `std::process::Command` module added to your project, you can now use it to execute Python scripts. Here is an example of how to execute a Python script using Rust:
use std::process::Command;
fn main() {
let output = Command::new("python")
.arg("my_script.py")
.output()
.expect("failed to execute process");
println!("{}", String::from_utf8_lossy(&output.stdout));
}
In this example, we are using the `Command::new` method to create a new command that will execute the `python` interpreter. We then use the `arg` method to specify the name of the Python script that we want to execute. Finally, we use the `output` method to execute the command and capture its output.
With this code, you can now execute Python scripts from within your Rust project. This can be useful if you need to integrate Python code into a larger Rust project, or if you need to take advantage of Rust’s performance and memory safety features in a Python project.
Equivalent of Python bin in Rust
In conclusion, the Rust programming language provides a powerful and efficient alternative to Python’s bin() function with its own built-in function called `format!()`. This function allows developers to easily convert integers to binary, octal, or hexadecimal formats with just a few lines of code. Additionally, Rust’s strong type system and memory safety features make it a reliable choice for building high-performance applications that require low-level control over memory management. Whether you’re a seasoned developer or just starting out, Rust’s bin function is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |