The Python dict function is used to create a dictionary object in Python. A dictionary is a collection of key-value pairs, where each key is unique and associated with a value. The dict function takes an iterable object as an argument and returns a dictionary object. The iterable object can be a list, tuple, set, or any other iterable object. The elements of the iterable object are used as keys in the dictionary, and the values are set to None by default. However, you can also provide values for the keys using the key-value syntax. The dict function is a built-in function in Python and is commonly used in data manipulation and analysis tasks. Keep reading below to learn how to python dict in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
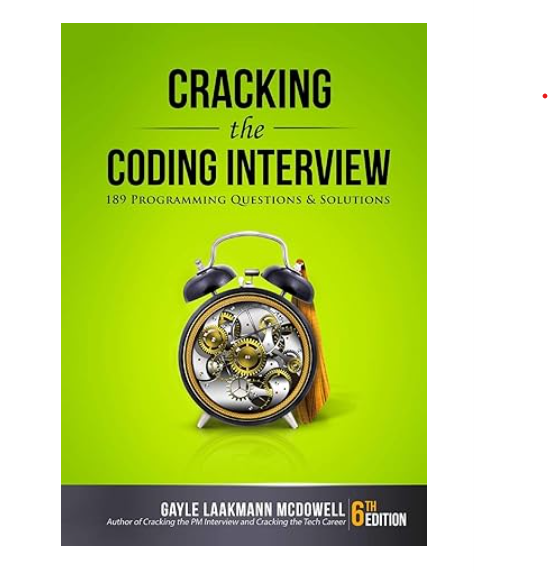
Python ‘dict’ in Go With Example Code
Python is a popular programming language that is widely used for various purposes, including web development, data analysis, and machine learning. One of the most useful data structures in Python is the dictionary, which allows you to store key-value pairs. If you are a Go developer, you may wonder how to implement a similar data structure in Go. In this blog post, we will explore how to create a dictionary-like data structure in Go.
In Go, the equivalent of a dictionary is a map. A map is a collection of key-value pairs, where each key is unique. You can create a map in Go using the make function, like this:
myMap := make(map[string]int)
This creates an empty map that can store string keys and integer values. You can add key-value pairs to the map using the following syntax:
myMap["key1"] = 1
myMap["key2"] = 2
You can also retrieve the value associated with a key using the following syntax:
value := myMap["key1"]
If the key does not exist in the map, the value returned will be the zero value for the value type. For example, if you try to retrieve a value for a key that does not exist in a map of integers, the value returned will be 0.
You can iterate over the keys and values in a map using a for loop, like this:
for key, value := range myMap {
fmt.Println(key, value)
}
This will print out all the key-value pairs in the map.
In conclusion, if you are a Python developer looking to implement a dictionary-like data structure in Go, you can use a map. A map is a collection of key-value pairs, where each key is unique. You can create a map using the make function, add key-value pairs using the square bracket syntax, retrieve values using the square bracket syntax, and iterate over the keys and values using a for loop.
Equivalent of Python dict in Go
In conclusion, the equivalent function of Python’s dict in Go is the map data type. Both Python’s dict and Go’s map are used to store key-value pairs, and they offer similar functionalities such as adding, deleting, and updating elements. However, there are some differences between the two, such as the syntax and the way they handle non-existent keys. Despite these differences, the map data type in Go is a powerful tool that can be used to solve a wide range of programming problems. Whether you are a Python developer looking to learn Go or a Go developer looking to expand your knowledge, understanding the map data type is essential for writing efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |