The Python dict function is used to create a dictionary object in Python. A dictionary is a collection of key-value pairs, where each key is unique and associated with a value. The dict function takes an iterable object as an argument and returns a dictionary object. The iterable object can be a list, tuple, set, or any other iterable object. The elements of the iterable object are used as keys in the dictionary, and the values are set to None by default. However, you can also provide values for the keys using the key-value syntax. The dict function is a built-in function in Python and is commonly used in data manipulation and analysis tasks.. Keep reading below to learn how to python dict in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
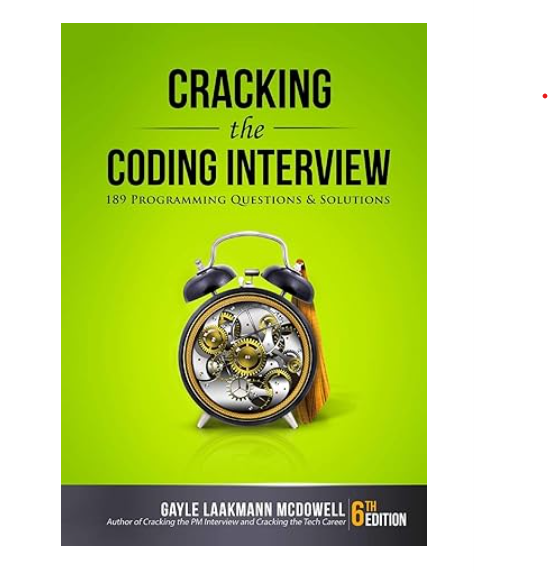
Python ‘dict’ in Java With Example Code
Python is a popular programming language that is widely used for various purposes. One of its most useful data structures is the dictionary, which allows you to store and retrieve key-value pairs efficiently. If you are a Java developer, you may be wondering how to implement a similar data structure in Java. In this blog post, we will explore how to create a Python dictionary in Java.
Java provides a similar data structure called a HashMap, which is part of the java.util package. A HashMap is a collection that stores key-value pairs, where each key is unique. To create a HashMap in Java, you can use the following syntax:
“`java
HashMap
“`
In this example, we are creating a HashMap that stores String keys and Integer values. You can replace these types with any other data types that you need.
To add a key-value pair to the HashMap, you can use the put() method:
“`java
myMap.put(“apple”, 1);
myMap.put(“banana”, 2);
myMap.put(“orange”, 3);
“`
In this example, we are adding three key-value pairs to the HashMap. The keys are “apple”, “banana”, and “orange”, and the values are 1, 2, and 3, respectively.
To retrieve a value from the HashMap, you can use the get() method:
“`java
int value = myMap.get(“banana”);
“`
In this example, we are retrieving the value associated with the key “banana”. The value variable will contain the value 2.
You can also check if a key exists in the HashMap using the containsKey() method:
“`java
if (myMap.containsKey(“apple”)) {
System.out.println(“The key ‘apple’ exists in the HashMap.”);
}
“`
In this example, we are checking if the key “apple” exists in the HashMap. If it does, we print a message to the console.
In conclusion, Java provides a similar data structure to Python’s dictionary called a HashMap. You can use the put() method to add key-value pairs, the get() method to retrieve values, and the containsKey() method to check if a key exists in the HashMap.
Equivalent of Python dict in Java
In conclusion, while Java does not have an exact equivalent to Python’s dict function, it does have similar data structures such as HashMap and LinkedHashMap that can be used to achieve similar functionality. These data structures allow for key-value pairs to be stored and accessed efficiently, making them useful for a variety of programming tasks. Additionally, Java’s object-oriented nature allows for more complex data structures to be created and manipulated as needed. Overall, while the syntax and implementation may differ, Java provides developers with powerful tools for working with key-value pairs and other data structures.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |