The Python dict function is used to create a dictionary object in Python. A dictionary is a collection of key-value pairs, where each key is unique and associated with a value. The dict function takes an iterable object as an argument and returns a dictionary object. The iterable object can be a list, tuple, set, or any other iterable object. The elements of the iterable object are used as keys in the dictionary, and the values are set to None by default. However, you can also provide values for the keys using the key-value syntax. The dict function is a built-in function in Python and is commonly used in data manipulation and analysis tasks. Keep reading below to learn how to python dict in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
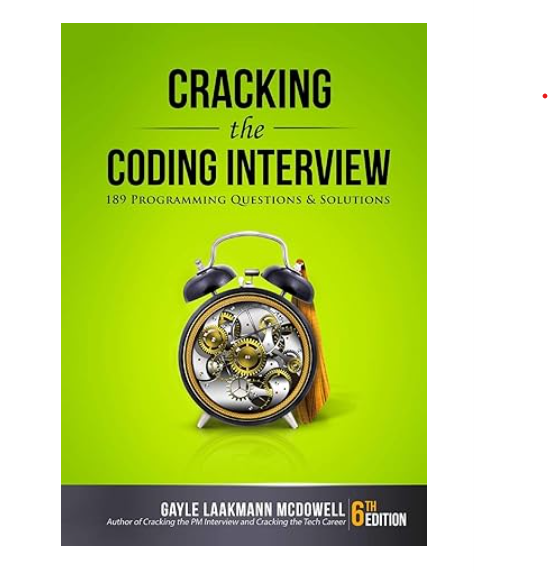
Python ‘dict’ in Rust With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. One of the most commonly used data structures in Python is the dictionary. A dictionary is a collection of key-value pairs, where each key is unique and maps to a corresponding value.
If you are a Rust developer, you may be wondering how to implement a dictionary in Rust. Fortunately, Rust provides a built-in data structure called a hash map that can be used to implement a dictionary.
To create a hash map in Rust, you can use the `HashMap` struct from the `std::collections` module. Here is an example of how to create a hash map in Rust:
use std::collections::HashMap;
fn main() {
let mut map = HashMap::new();
map.insert("key1", "value1");
map.insert("key2", "value2");
map.insert("key3", "value3");
println!("{:?}", map);
}
In this example, we create a new hash map using the `HashMap::new()` method. We then insert three key-value pairs into the map using the `insert()` method. Finally, we print the contents of the map using the `println!()` macro.
To access a value in the hash map, you can use the `get()` method and provide the key as an argument. Here is an example:
use std::collections::HashMap;
fn main() {
let mut map = HashMap::new();
map.insert("key1", "value1");
map.insert("key2", "value2");
map.insert("key3", "value3");
let value = map.get("key2");
match value {
Some(v) => println!("The value of key2 is {}", v),
None => println!("Key not found"),
}
}
In this example, we use the `get()` method to retrieve the value associated with the key “key2”. We then use a `match` statement to handle the case where the key is not found in the map.
In conclusion, Rust provides a built-in hash map data structure that can be used to implement a dictionary. By using the `HashMap` struct from the `std::collections` module, you can easily create, insert, and retrieve key-value pairs in Rust.
Equivalent of Python dict in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to work with dictionaries through its built-in HashMap data structure. The HashMap in Rust is similar to the Python dict function, allowing developers to store and retrieve key-value pairs with ease. With its strong type system and memory safety features, Rust provides a reliable and secure way to work with dictionaries in your code. Whether you are a seasoned developer or just starting out, Rust’s HashMap is a great tool to have in your programming arsenal. So, if you’re looking for a fast and reliable way to work with dictionaries in Rust, be sure to check out the HashMap function.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |