The divmod() function in Python takes two arguments and returns a tuple containing the quotient and remainder of the division operation. The first argument is the dividend and the second argument is the divisor. The function performs integer division and returns the quotient as the first element of the tuple and the remainder as the second element. This function is useful when you need to perform both division and modulo operations on the same pair of numbers, as it saves you from having to perform two separate calculations.. Keep reading below to learn how to python divmod in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
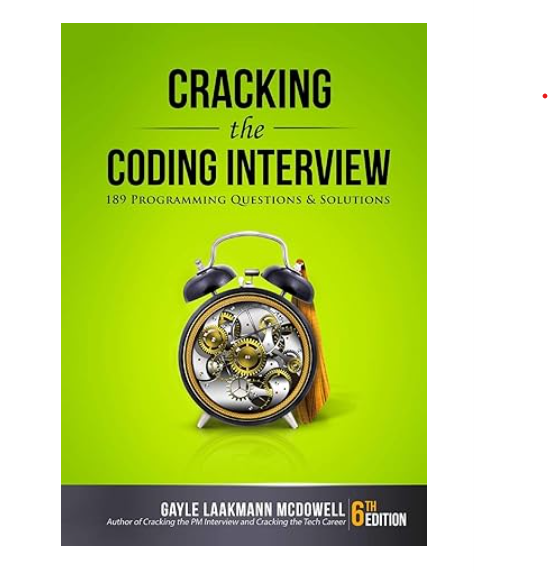
Python ‘divmod’ in C# With Example Code
Python’s built-in function `divmod()` is a handy tool for performing both division and modulo operations at the same time. But what if you’re working in C# and need to replicate this functionality? Fortunately, C# has its own built-in method for performing both operations simultaneously: `Math.DivRem()`.
To use `Math.DivRem()`, simply pass in the dividend, divisor, and an out parameter for the remainder. The method will return the quotient, and the remainder will be stored in the out parameter. Here’s an example:
“`
int dividend = 10;
int divisor = 3;
int quotient, remainder;
quotient = Math.DivRem(dividend, divisor, out remainder);
Console.WriteLine(“Quotient: ” + quotient);
Console.WriteLine(“Remainder: ” + remainder);
“`
This will output:
“`
Quotient: 3
Remainder: 1
“`
As you can see, `Math.DivRem()` performs both the division and modulo operations in one step, just like `divmod()` in Python.
One thing to note is that `Math.DivRem()` returns the same sign as the dividend, whereas Python’s `divmod()` returns the same sign as the divisor. So if you’re working with negative numbers, you may need to adjust accordingly.
Overall, `Math.DivRem()` is a useful tool to have in your C# arsenal when you need to perform both division and modulo operations at the same time.
Equivalent of Python divmod in C#
In conclusion, the divmod function in Python is a useful tool for performing division and modulus operations simultaneously. While C# does not have an equivalent built-in function, it is possible to create a similar function using basic arithmetic operations. By using integer division and modulus operators, we can achieve the same result as the divmod function in Python. With a little bit of coding knowledge, we can easily implement this function in C# and use it in our programs. Overall, the divmod function is a great example of how programming languages can differ in their built-in functions, but with a little creativity, we can achieve the same results across different languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |