The divmod() function in Python takes two arguments and returns a tuple containing the quotient and remainder of the division operation. The first argument is the dividend and the second argument is the divisor. The function performs integer division and returns the quotient as the first element of the tuple and the remainder as the second element. This function is useful when you need to perform both division and modulo operations on the same pair of numbers, as it saves you from having to perform two separate calculations. Keep reading below to learn how to python divmod in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
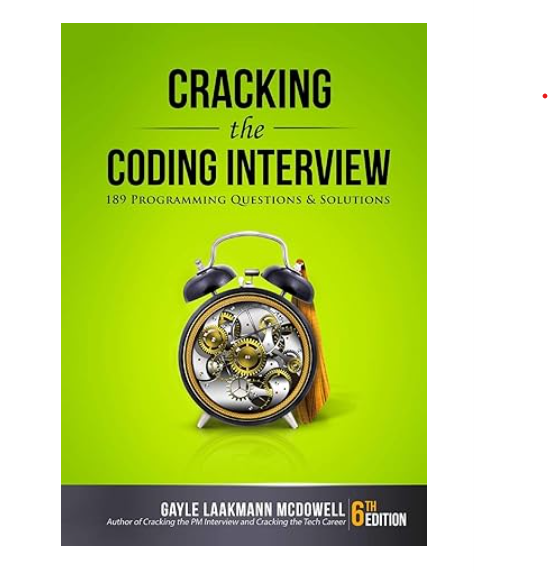
Python ‘divmod’ in PHP With Example Code
Python’s built-in function `divmod()` is a useful tool for performing division and modulus operations simultaneously. While PHP does not have a direct equivalent to `divmod()`, it is possible to replicate its functionality using a combination of the `floor()` and `%` operators.
To use `divmod()` in Python, you simply pass in two numbers as arguments and it returns a tuple containing the quotient and remainder of the division operation. For example:
result = divmod(10, 3)
print(result) # Output: (3, 1)
To replicate this functionality in PHP, you can use the `floor()` function to get the quotient and the `%` operator to get the remainder. Here’s an example:
function divmod($a, $b) {
$quotient = floor($a / $b);
$remainder = $a % $b;
return array($quotient, $remainder);
}
$result = divmod(10, 3);
print_r($result); // Output: Array ( [0] => 3 [1] => 1 )
In this example, the `divmod()` function takes two arguments, `$a` and `$b`, and returns an array containing the quotient and remainder of the division operation. The `floor()` function is used to get the quotient, and the `%` operator is used to get the remainder.
By using this `divmod()` function, you can replicate the functionality of Python’s built-in `divmod()` function in PHP.
Equivalent of Python divmod in PHP
In conclusion, the divmod function in Python is a useful tool for performing division and modulus operations simultaneously. While PHP does not have an equivalent built-in function, it is possible to achieve the same result using the floor and fmod functions. By combining these functions, we can perform division and modulus operations in a single line of code, just like the divmod function in Python. With a little bit of creativity and knowledge of PHP’s built-in functions, we can achieve the same functionality as the divmod function in Python.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |