The Python enumerate function is a built-in function that allows you to iterate over a sequence while keeping track of the index of the current item. It takes an iterable object as an argument and returns an iterator that generates tuples containing the index and the corresponding item from the iterable. The first element of the tuple is the index, starting from 0, and the second element is the item from the iterable. This function is useful when you need to access both the index and the value of each item in a sequence, such as a list or a string. Keep reading below to learn how to python enumerate in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
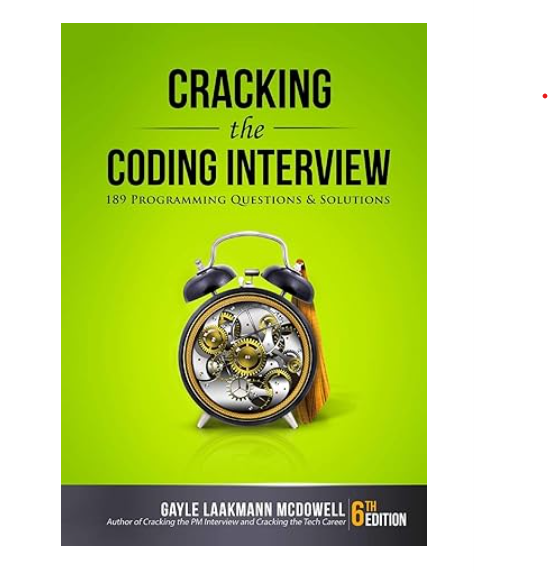
Python ‘enumerate’ in Go With Example Code
Python’s `enumerate` function is a useful tool for iterating over a list or other iterable object while keeping track of the index of each item. If you’re working in Go and looking for a similar functionality, you’re in luck! Go has a built-in `range` function that can be used to achieve the same result.
To use `range` in a similar way to `enumerate`, you can use the `for` loop syntax and assign both the index and value of each item to variables. Here’s an example:
fruits := []string{"apple", "banana", "cherry"}
for i, fruit := range fruits {
fmt.Println(i, fruit)
}
In this example, `i` represents the index of each item in the `fruits` slice, and `fruit` represents the value of each item. The `fmt.Println` statement prints out both the index and value for each item.
You can also use `range` with maps to iterate over key-value pairs. Here’s an example:
ages := map[string]int{"Alice": 25, "Bob": 30, "Charlie": 35}
for name, age := range ages {
fmt.Println(name, age)
}
In this example, `name` represents the key of each key-value pair in the `ages` map, and `age` represents the value. The `fmt.Println` statement prints out both the key and value for each pair.
Using `range` in Go is a powerful tool for iterating over iterable objects while keeping track of the index or key of each item. With a little practice, you’ll be using it like a pro in no time!
Equivalent of Python enumerate in Go
In conclusion, the equivalent of Python’s enumerate function in Go is the for-range loop. While the syntax may be different, the functionality is essentially the same. The for-range loop allows us to iterate over a collection of elements and access both the index and value of each element. This makes it a powerful tool for working with arrays, slices, and maps in Go. By using the for-range loop, we can simplify our code and make it more readable, while still achieving the same results as we would with Python’s enumerate function. Overall, the for-range loop is a valuable feature of Go that every developer should be familiar with.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |