The Python enumerate function is a built-in function that allows you to iterate over a sequence while keeping track of the index of the current item. It takes an iterable object as an argument and returns an iterator that generates tuples containing the index and the corresponding item from the iterable. The first element of the tuple is the index, starting from 0, and the second element is the item from the iterable. This function is useful when you need to access both the index and the value of each item in a sequence, such as a list or a string. Keep reading below to learn how to python enumerate in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
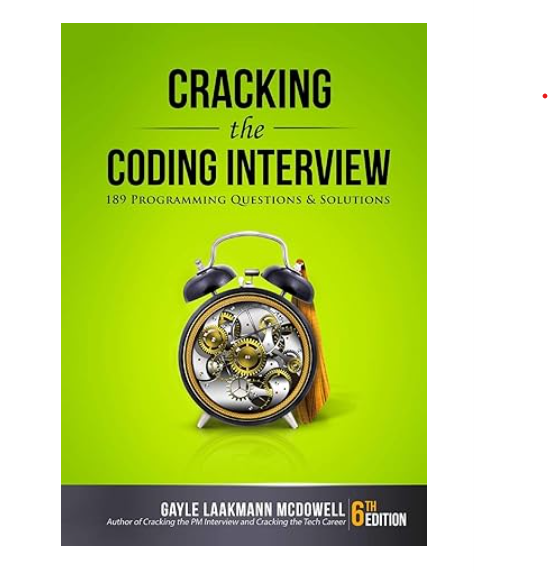
Python ‘enumerate’ in Kotlin With Example Code
Python’s `enumerate` function is a useful tool for iterating over a list or other iterable while keeping track of the index of the current item. Kotlin doesn’t have an exact equivalent to Python’s `enumerate`, but there are a few ways to achieve similar functionality.
One option is to use the `withIndex()` function, which is available on any iterable in Kotlin. This function returns an `Iterable` of `IndexedValue` objects, which contain both the index and the value of each item in the original iterable. Here’s an example:
val myList = listOf("apple", "banana", "cherry")
for ((index, value) in myList.withIndex()) {
println("The element at index $index is $value")
}
This code will output:
The element at index 0 is apple
The element at index 1 is banana
The element at index 2 is cherry
Another option is to use a `forEachIndexed` function, which is available on arrays and lists in Kotlin. This function takes a lambda with two parameters: the index and the value of each item. Here’s an example:
val myList = listOf("apple", "banana", "cherry")
myList.forEachIndexed { index, value ->
println("The element at index $index is $value")
}
This code will output the same result as the previous example.
In summary, while Kotlin doesn’t have an exact equivalent to Python’s `enumerate`, the `withIndex()` function and `forEachIndexed` function can be used to achieve similar functionality.
Equivalent of Python enumerate in Kotlin
In conclusion, the equivalent of Python’s enumerate function in Kotlin is the withIndex function. This function allows us to iterate over a collection while also accessing the index of each element. It is a convenient and efficient way to perform operations on a collection while keeping track of the index. With the withIndex function, Kotlin developers can easily implement the same functionality as the Python enumerate function. Overall, Kotlin’s withIndex function is a powerful tool that can simplify the code and make it more readable.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |